Делал такую лабу) Вот код класса многчлена. Так же есть готовые формочки и тп, а также реализация на двузвязном циклическом списке
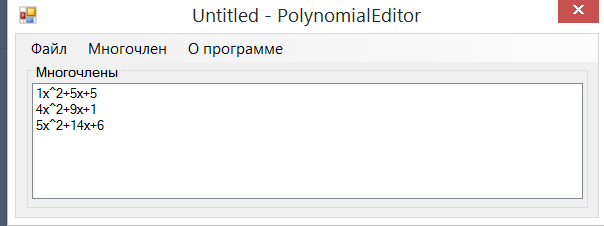
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Text.RegularExpressions;
namespace WindowsFormsApplication1
{
public class Polinom
{
private Node head;
//элементарное кольцо
public Polinom()
{
head = new Node();
head.Next = head;
head.Prev = head;
}
//инициализируем строкой
public Polinom(string PolynomString)
{
head = new Node();
head.Next = head;
head.Prev = head;
Parse(PolynomString);
}
//создание мночлена из строки
public void Parse(string PolynomString)
{
head.Next = head;
head.Prev = head;
Regex polynomRegExp = new Regex("^[+-]?\\d*(?:x(?:\\^\\d+)?)?(?:[+-]\\d*(?:x(?:\\^\\d+)?)?)*$");
Regex termRegExp = new Regex("[+-]?\\d*(?:x(?:\\^\\d+)?)?");
if (polynomRegExp.IsMatch(PolynomString))
{
MatchCollection terms = termRegExp.Matches(PolynomString);
foreach (Match term in terms)
Insert(term.ToString());
}
else
throw new FormatException();
}
//вставка члена
public void Insert(int degree, int coef)
{
if (coef != 0)
{
Node term = head.Next;
while ((term != head) && (term.Degree < degree))
term = term.Next; //ищем место вставки
if (degree != term.Degree || term == head) //вставка слева от найденного
{
Node newTerm = new Node(degree, coef);
newTerm.Next = term;
newTerm.Prev = term.Prev;
term.Prev.Next = newTerm;
term.Prev = newTerm;
}
else //если степень уже существует - складываем коэфициенты
{
term.Coef += coef;
if (term.Coef == 0) //поглощение членов
Delete(term.Degree);
}
}
}
//вставка члена из строки
public void Insert(string termString)
{
int degree, coef;
Node.Parse(termString, out degree, out coef);
if (coef != 0)
Insert(degree, coef);
}
//удаление члена заданной степени
public void Delete(int degree)
{
Node term = head.Next;
while ((term != head) && (term.Degree != degree))
term = term.Next;
if (term != head)
{
term.Prev.Next = term.Next;
term.Next.Prev = term.Prev;
}
else
throw new IndexOutOfRangeException(); //если не нашли
}
//возвращает строковое представление многочлена
override public string ToString()
{
StringBuilder Expr = new StringBuilder();
Node term = head.Next;
while (term != head)
{
if ((Expr.Length != 0) && (Expr[0] != '-'))
Expr.Insert(0, '+'); //знак плюса перед членом
Expr.Insert(0, term.ToString());
term = term.Next;
}
return Expr.ToString();
}
//сложение
public Polinom Addition(Polinom polinom)
{
Polinom result = new Polinom(polinom.ToString());
Node term = head.Next;
while (term != head)
{
result.Insert(term.Degree, term.Coef);
term = term.Next;
}
return result;
}
public static Polinom operator +(Polinom p1, Polinom p2)
{
return p1.Addition(p2);
}
}
}
//класс члена
public class Node
{
private int stepen; //степень члена
private int a; //коэффициент члена
private Node link; // поле связи
//свойства
public int stepen
{
get { return stepen; }
set { stepen = value; }
}
public int A
{
get { return a; }
set { a = value; }
}
public Node Link
{
get { return link; }
set { link = value; }
}
//конструкторы
public Node()
{
}
public Node(int degree, int coef)
{
stepen = degree;
a = coef;
Link = null;
}
public Node(int stepen, int a, Node link)
{
Stepen = stepen;
A = a;
Link = link;
}
//строковое представление члена
public override string ToString()
{
StringBuilder Expr = new StringBuilder();
if (stepen == 0)
Expr.Insert(0, a);
else if (stepen == 1)
Expr.Insert(0, String.Format("{0}x", a));
else
Expr.Insert(0, String.Format("{0}x^{1}", a, stepen));
return Expr.ToString();
}
//парсит строку члена в параметры stepen и a
public static void Parse(string termString, out int degree, out int coef)
{
if (termString != "")
{
Regex termRegExp = new Regex("^([+-]?\\d*)(?:(x)(?:\\^(\\d+))?)?$");
if (termRegExp.IsMatch(termString))
{
Match match = termRegExp.Match(termString);
string coefMatch = match.Groups[1].ToString();
string xMatch = match.Groups[2].ToString();
string degreeMatch = match.Groups[3].ToString();
if (coefMatch == "+" || coefMatch == "")
coef = 1;
else if (coefMatch == "-")
coef = -1;
else
coef = Int32.Parse(coefMatch);
if (degreeMatch != "")
degree = Int32.Parse(degreeMatch);
else if (xMatch == "x")
degree = 1;
else
degree = 0;
}
else
throw new FormatException();
}
else
{
coef = 0;
degree = 0;
}
}
}