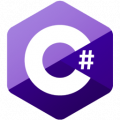
C#
3
Вклад в тег
<Window x:Class="WpfApplication1.MainWindow"
xmlns="<a href="http://schemas.microsoft.com/winfx/2006/xaml/presentation">http://schemas.microsoft.com/winfx/2006/xaml/presentation</a>"
xmlns:x="<a href="http://schemas.microsoft.com/winfx/2006/xaml">http://schemas.microsoft.com/winfx/2006/xaml</a>"
xmlns:wpfApplication1="clr-namespace:WpfApplication1"
Title="MainWindow" Height="350" Width="525">
<Window.Resources>
<ResourceDictionary>
<wpfApplication1:ConverterNull x:Key="ConverterNull"/>
</ResourceDictionary>
</Window.Resources>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="30"></RowDefinition>
<RowDefinition Height="5"></RowDefinition>
<RowDefinition Height="30"></RowDefinition>
</Grid.RowDefinitions>
<ComboBox Name ="cmb" DisplayMemberPath="Value" SelectedValuePath = "id" ></ComboBox>
<TextBox Name="tb" Grid.Row="2" Text="{Binding ElementName=cmb, Path=SelectedItem.Value,Mode=TwoWay,UpdateSourceTrigger=PropertyChanged,Converter={StaticResource ConverterNull}}"></TextBox>
</Grid>
</Window>
<Window.Resources>
<ResourceDictionary>
<wpfApplication1:ConverterNull x:Key="ConverterNull"/>
</ResourceDictionary>
</Window.Resources>
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Windows;
using System.Windows.Data;
namespace WpfApplication1
{
public partial class MainWindow : Window
{
private List<Info> info;
public MainWindow()
{
InitializeComponent();
info = new List<Info>
{
new Info("a"),
new Info("b"),
new Info("c")
};
cmb.ItemsSource = info;
}
}
public class Info
{
public Guid id { get; private set; }
public string Value { get; set; }
public Info(String val)
{
id = Guid.NewGuid();
Value = val;
}
}
sealed class ConverterNull : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
return value;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
return value == null ? null : String.IsNullOrWhiteSpace(value.ToString()) ? null : value;
}
}
}