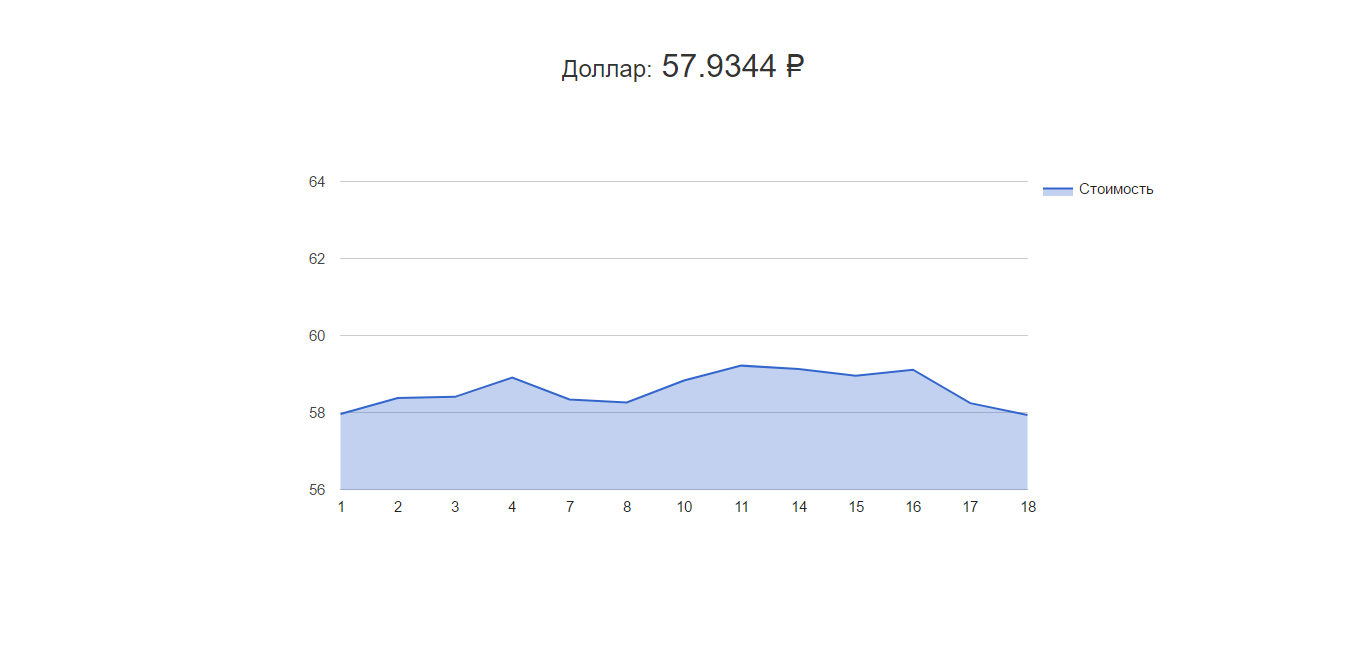
Делал на скорую руку
<?php
function get($url) {
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt ($ch, CURLOPT_TIMEOUT, 60);
curl_setopt ($ch, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt ($ch, CURLOPT_FAILONERROR, true);
$data = curl_exec($ch);
echo curl_error($ch);
curl_close($ch);
return $data;
}
function url() {
$to = date("d/m/Y");
$d = date("d");
$m = date("m");
$y = date("Y") - 1;
$from = "$d/$m/$y";
$url = "http://www.cbr.ru/scripts/XML_dynamic.asp?date_req1=$from&date_req2=$to&VAL_NM_RQ=R01235";
return get($url);
}
function today($array) {
$max = count($array["Record"]) - 1;
$price = str_replace(",", ".", $array["Record"][$max]["Value"]);
return $price;
}
$content = url();
$xml = simplexml_load_string($content);
$json = json_encode($xml);
$array = json_decode($json, TRUE);
?>
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript" src="https://www.gstatic.com/charts/loader.js"></script>
<script type="text/javascript">
google.charts.load('current', {'packages':['corechart']});
google.charts.setOnLoadCallback(drawChart);
function drawChart() {
var data = google.visualization.arrayToDataTable([
["Дата", "Стоимость"],
<?
for($i = 0; $i < count($array["Record"]); $i++) {
$date = date("d.m.Y", strtotime($array["Record"][$i]["@attributes"]["Date"]));
$price = str_replace(",", ".", $array["Record"][$i]["Value"]);
if($i == count($array["Record"]) - 1) {
echo "['$date', $price]\n";
} else {
echo "['$date', $price],\n";
}
}
?>
]);
var options = {
vAxis: {minValue: <?=today($array)*1.1?>}
};
var chart = new google.visualization.AreaChart(document.getElementById("dollar"));
chart.draw(data, options);
}
</script>
<style>
body {
width: 75vw;
margin: 3em auto;
font-family: Arial, sans-serif;
font-size: 16px;
}
.title {
text-align: center;
color: #333;
width: 100%;
font-size: 2em;
}
.dollar:before {
content: "Доллар: ";
font-size: 0.75em;
}
.dollar:after {
content: " ₽";
font-size: 0.75em;
}
</style>
</head>
<body>
<div class="title dollar"><?=today($array)?></div>
<div id="dollar" style="width: 100%; height: 500px;"></div>
</body>
</html>