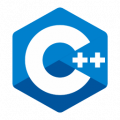
C++
- 22 ответа
- 0 вопросов
15
Вклад в тег
#include <iostream>
using namespace std;
int main() {
cout << "2 << 1 == " << (0b010 << 1); // 0b100
return 0;
}
#include <iostream>
using namespace std;
struct Foo {
Foo& operator<<(int x) {
cout << "Integer: " << x << '\n';
return *this;
}
Foo& operator<<(const char* str) {
cout << "String: " << str << '\n';
return *this;
}
};
int main() {
Foo foo;
foo << 3; // Integer: 3
foo.operator<<("Hello world"); // String: Hello world
return 0;
}
#include <iostream>
using namespace std;
using esp_spp_cb_event_t = int;
using esp_spp_cb_param_t = void;
void btCallback(esp_spp_cb_event_t event, esp_spp_cb_param_t *param) {
cout << "btCallback" << endl;
}
class MyBt {
public:
typedef void (*callback_type)(esp_spp_cb_event_t, esp_spp_cb_param_t *);
void register_callback(callback_type cb) {
cb(0, nullptr);
}
};
int main()
{
MyBt bt;
bt.register_callback(btCallback);
return 0;
}
p = p2
мы выполняем неявное преобразование встроенных типов, для которых в стандарте не описаны правила преобразования. То есть такое преобразование не гарантировано, но может быть реализовано компилятором, например#include <type_traits>
using namespace std;
int main() {
static_assert(std::is_convertible<int(*)[2], int(*)[]>::value, "Non-convertible");
return 0;
}