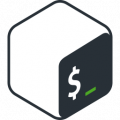
bash
- 2 ответа
- 0 вопросов
0
Вклад в тег
#!/bin/sh
for file in \
/etc/squid/URLs/accessed/access.sorted \
/etc/squid/URLs/accessed/oper_kass_url \
/etc/squid/URLs/denied/deny.url.uniq \
;
do
cp $file /home/security/urls/
done
for file in \
/etc/squid/groups/full.dom \
/etc/squid/groups/limit.dom \
/etc/squid/groups/oper_kass.dom \
/etc/squid/groups/unlim.dom \
;
do
cp $file /home/security/groups/
done
for file in \
/etc/squid/squid.conf \
;
do
cp $file /home/security/urls/
done
sub adsp {
my $rand = int rand @arrset;
my $rand_num = $arrset[$rand];
$rand_num =~ /^(\d+)_(\d+)$/;
my $buf = $1;
my $buf2 = $2;
@arrset = grep { !/^\d+_$buf$/ } @arrset;
@arrset = grep { !/^\d+_$buf2$/ } @arrset;
@arrset = grep { !/^${buf}_\d+$/ } @arrset;
@arrset = grep { !/^${buf2}_\d+$/ } @arrset;
return $rand_num;
}
use strict;
use warnings;
# квадрат расстояния между двумя точками
def length2(a, b):
return (a[0] - b[0])**2 + (a[1] - b[1])**2
# подобны ли 2 треугольника, заданные отсортированными массивами длин сторон
def is_sim(sample, triangle):
if sample[0] * triangle[1] != sample[1] * triangle[0]:
return False
elif sample[0] * triangle[2] != sample[2] * triangle[0]:
return False
elif sample[1] * triangle[2] != sample[2] * triangle[1]:
return False
else:
return True
# преобразование массивов координат в массивы длин сторон
def make_triangles(v):
triangles = []
for i in v:
a2 = length2(i[0], i[1])
b2 = length2(i[0], i[2])
c2 = length2(i[1], i[2])
triangle = [a2, b2, c2]
triangle.sort()
triangles.append(triangle)
return triangles
# основной код
triangles = make_triangles(v)
for i in range(1, len(triangles) ):
if is_sim(triangles[0], triangles[i]):
print "found similar trinangle: %s" % v[i]