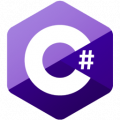
C#
- 30 ответов
- 0 вопросов
18
Вклад в тег
In Java, all non-static methods are by default "virtual functions." Only methods marked with the keyword final, which cannot be overridden, along with private methods, which are not inherited, are non-virtual.
void Main()
{
new B("name");
}
class A
{
public A()
{
Method();
}
protected virtual void Method()
{
}
}
class B : A
{
private string Property { get; set; }
public B(string value)
{
Property = value;
}
protected override void Method()
{
Console.WriteLine(Property.Length);
}
}
<Window x:Class="WpfApplication1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApplication1"
mc:Ignorable="d"
Title="MainWindow" Height="350" Width="525">
<Button Click="Button_Click">
<ToggleButton Click="ToggleButton_Click" />
</Button>
</Window>
private void Button_Click(object sender, RoutedEventArgs e)
{
MessageBox.Show("Button clicked");
}
private void ToggleButton_Click(object sender, RoutedEventArgs e)
{
MessageBox.Show("ToggleButton clicked");
e.Handled = true;
}