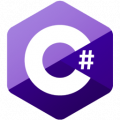
C#
- 64 ответа
- 0 вопросов
47
Вклад в тег
class TextBoxWithPlaceholder : TextBox
{
public string Placeholder { get; set; }
protected override void OnCreateControl()
{
base.OnCreateControl();
if (!DesignMode)
{
Text = Placeholder;
}
}
protected override void OnLostFocus(EventArgs e)
{
base.OnLostFocus(e);
if (Text.Equals(string.Empty))
{
Text = Placeholder;
}
}
protected override void OnGotFocus(EventArgs e)
{
base.OnGotFocus(e);
if (Text.Equals(Placeholder))
{
Text = string.Empty;
}
}
}
var client = new POPClient();
client.Connect("pop.gmail.com", 995, true);
client.Authenticate("admin@bendytree.com", "YourPasswordHere");
var count = client.GetMessageCount();
Message message = client.GetMessage(count);
Console.WriteLine(message.Headers.Subject);
dynamic myDinamic = GetDynamic();
myDynamic.SomeMethod();
public class MyNumeric<T> where T : struct, IComparable<T>, IEquatable<T>, IConvertible
{
public static readonly T MaxValue = ReadStaticField("MaxValue");
public static readonly T MinValue = ReadStaticField("MinValue");
private static T ReadStaticField(string name)
{
FieldInfo field = typeof(T).GetField(name, BindingFlags.Public | BindingFlags.Static);
if (field == null)
{
throw new InvalidOperationException("Нечисловой тип: " + typeof(T).Name);
}
return (T)field.GetValue(null);
}
}
Console.WriteLine(MyNumeric<int>.MaxValue);
Console.WriteLine(MyNumeric<float>.MinValue);