Есть такой класс
use Yii;
use yii\db\ActiveRecord;
use yii\behaviors\TimestampBehavior;
use yii\db\Expression;
/**
* This is the model class for table "game_zones".
*
* @property int $id
* @property string $name
* @property string $status
* @property string $start_of_set
* @property string $start_of_game
* @property string $end_of_game
*/
class GameZones extends ActiveRecord
{
const ZONE_STATUS_SET = 'set';
const ZONE_STATUS_GAME = 'game';
const ZONE_STATUS_GAME_OVER = 'game_over';
/**
* {@inheritdoc}
*/
public static function tableName()
{
return 'game_zones';
}
/**
* {@inheritdoc}
*/
public function rules()
{
return [
[['name', 'status', 'start_of_set'], 'required'],
[['status'], 'string'],
[['start_of_set', 'start_of_game', 'end_of_game'], 'safe'],
[['name'], 'string', 'max' => 3],
];
}
public function __construct(array $config = [])
{
$this->name = self::makeName();
$this->status = self::ZONE_STATUS_SET;
parent::__construct($config);
}
public function behaviors()
{
return [
[
'class' => TimestampBehavior::class,
'attributes' => [
ActiveRecord::EVENT_BEFORE_INSERT => ['start_of_set'],
],
'value' => new Expression('NOW()'),
],
];
}
/**
* {@inheritdoc}
*/
public function attributeLabels()
{
return [
'id' => 'ID',
'name' => 'Name',
'status' => 'Status',
'start_of_set' => 'Start Of Set',
'start_of_game' => 'Start Of Game',
'end_of_game' => 'End Of Game',
];
}
public static function makeName()
{
$name = 'A01';
$lastZone = self::find()->orderBy(['name' => SORT_DESC])->one();
if ($lastZone) {
$lastName = $lastZone->name;
if (preg_replace('/\D/', '', $lastName) != 99) {
$name = ++$lastName;
} else {
$prefix = preg_replace('/\d/', '', $lastName);
$name = ++$prefix . '01';
}
}
return $name;
}
}
При вызове makeName(), получается бесконечно повторяющийся запрос к БД
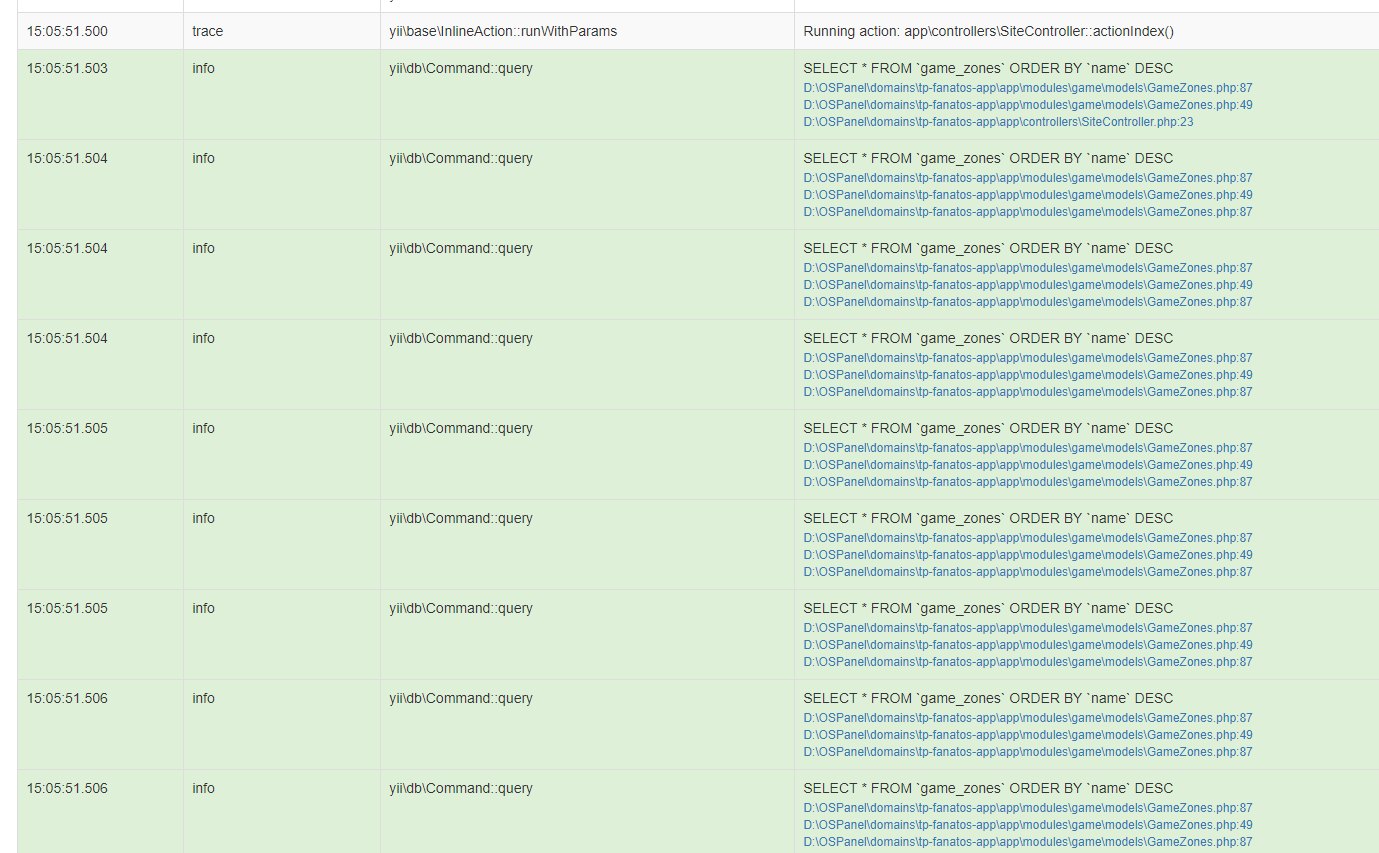
В чем я туплю? Как это можно исправить?