Отладчик помечает код как недостижимый? Из-за этого обмен по сети не работает.
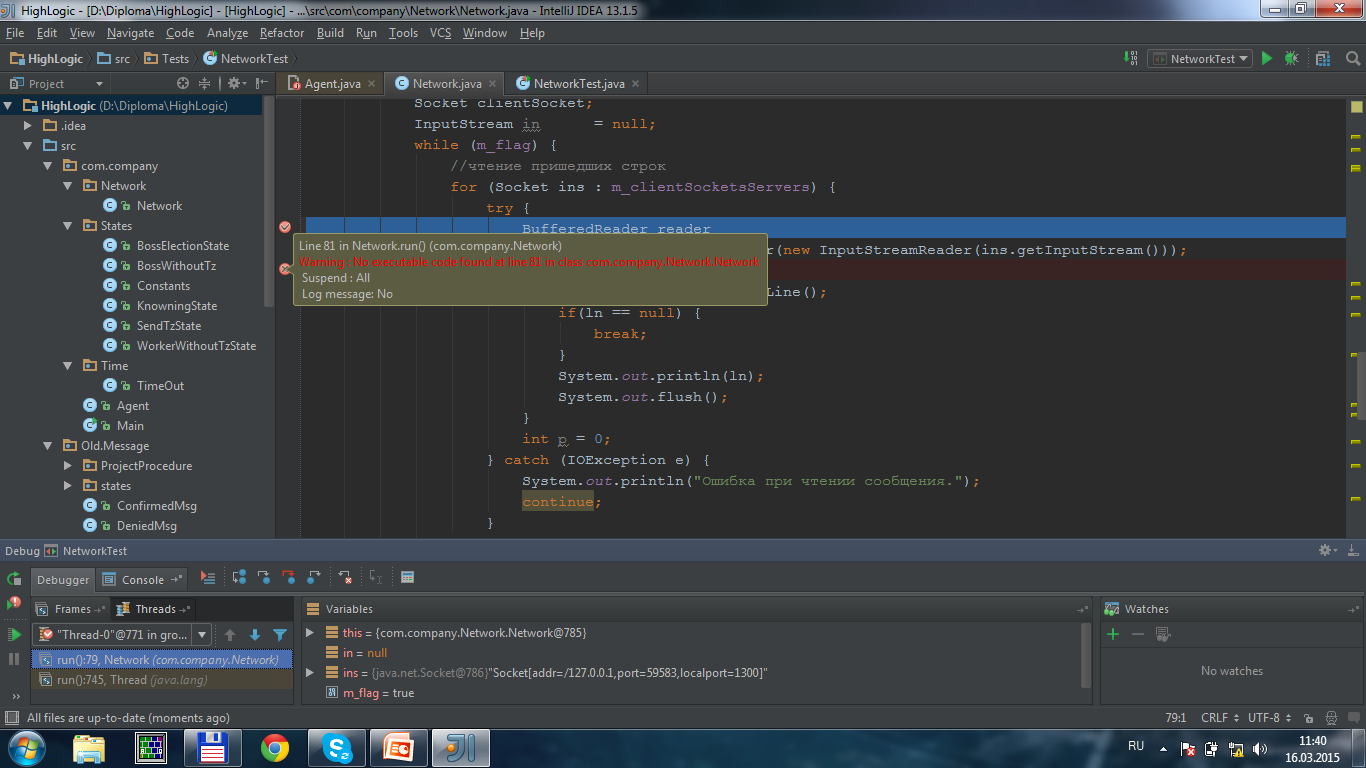
package com.company.Network;
import java.io.*;
import java.net.*;
import java.util.LinkedList;
import Old.Message.Message;
import Tubes.Tube;
/**
* @class Network
* класс-поток для работы с сетью
*/
public class Network implements Runnable {
private Tube m_in;
private Tube m_out;
private final int m_port = 1300;
private ServerSocket m_serverSocket;
private int m_netType;
private boolean m_flag;
LinkedList<Socket> m_clientSocketsServers;
Socket m_clientSocket;
public Network() {
m_netType = -1;
m_flag = true;
m_clientSocketsServers
= new LinkedList<Socket>();
}
public void stayServer() {
m_netType = 0;
try {
m_serverSocket = new ServerSocket(m_port, 0,
InetAddress.getByName("localhost"));
} catch (IOException e) {
System.out.println(e.toString());
}
}
public void stayClient() {
m_netType = 1;
while (m_flag) {
try {
m_clientSocket = new Socket(InetAddress.getByName("localhost"), m_port);
break;
} catch (UnknownHostException e) {
System.out.println("Неизвестныйхост: " + "localhost");
continue;
} catch (IOException e) {
System.out.println("Ошибка ввода/вывода при создании сокета " + "localhost"
+ ":" + m_port);
continue;
}
}
}
public void stopNet() {
m_flag = false;
}
///метод обмена информацией
@Override
public void run() {
if(m_netType == -1) {
return;
}
//as server
if(m_netType == 0) {
Socket clientSocket;
InputStream in = null;
while (m_flag) {
//чтение пришедших строк
for (Socket ins : m_clientSocketsServers) {
try {
BufferedReader reader
= new BufferedReader(new InputStreamReader(ins.getInputStream()));
while (true) {
String ln = reader.readLine();
if(ln == null) {
break;
}
System.out.println(ln);
System.out.flush();
}
int p = 0;
} catch (IOException e) {
System.out.println("Ошибка при чтении сообщения.");
continue;
}
}
try {
clientSocket = m_serverSocket.accept();
m_clientSocketsServers.add(clientSocket);
} catch (IOException e) {
continue;
}
}
}
//as client
if(m_netType == 1) {
OutputStream out = null;
PrintWriter writter = null;
try {
out = m_clientSocket.getOutputStream();
writter = new PrintWriter(out);
} catch (IOException e) {
System.out.println("Не удалось получить поток вывода.");
}
for(int i = 0; i < 10; i++) {
writter.write(i);
writter.flush();
}
}
}
}