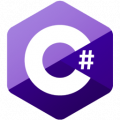
C#
4
Вклад в тег
private string _content;
public string content
{
get => _content;
set { OnPropertyChanged(ref _content, value); }
}
<Label Content="{Binding content}" Foreground="{Binding fontColor}" FontSize="{Binding fontSize}"/>
private RelayCommand _mouseEnterCommand;
/// <summary>
/// Команда входа мышки в поле контролера
/// </summary>
public RelayCommand MouseEnterCommand
{
get { return _mouseEnterCommand; }
set
{
OnPropertyChanged(ref _mouseEnterCommand, value);
}
}
private RelayCommand _mouseLeaveCommand;
/// <summary>
/// Команда покидания мышки поля контролера
/// </summary>
public RelayCommand MouseLeaveCommand
{
get { return _mouseLeaveCommand; }
}
private RelayCommand _mouseDownCommand;
/// <summary>
/// Команда щелчка мышки
/// </summary>
public RelayCommand MouseDownCommand
{
get { return _mouseDownCommand; }
}
event Action _mouseEnter;
event Action _mouseLeave;
void MouseEnterEventMethod()
{
fontColor = fontColorMouseEnter;
}
void MouseLeaveEventMethod()
{
fontColor = fontColorNormal;
}
private void MouseEnter()
{
this._mouseEnter.Invoke();
}
private void MouseLeave()
{
this._mouseLeave.Invoke();
}
public void MouseEnter(object param)
{
MouseEnter();
}
public void MouseLeave(object param)
{
MouseLeave();
}
public AButton(string _content)
{
content = _content;
_mouseEnter += this.MouseEnterEventMethod;
_mouseLeave += this.MouseLeaveEventMethod;
this._mouseEnterCommand = new RelayCommand(MouseEnter);
this._mouseLeaveCommand = new RelayCommand(MouseLeave);
}
class ButtonStyleA : AButton
{
public ButtonStyleA(string _content) : base()
{
content = _content;
base.fontColor = "#FF5733";
base.fontColorNormal = "#FF5733";
base.fontColorMouseEnter = "#61FF33";
fontSize = 16;
}
}
class ButtonStyleB : AButton
{
public ButtonStyleB(string _content) : base()
{
content = _content;
fontColor = "#33B5FF";
fontColorNormal = "#33B5FF";
fontColorMouseEnter = "#E933FF";
fontSize = 12;
}
}
public class MenuButtonViewModel
{
public AButton MenuButton { get; set; }
public MenuButtonViewModel(AButton menuButton)
{
MenuButton = menuButton;
}
}
xmlns:u="clr-namespace:WpfMvvmDemo.Services"
<Grid u:MouseBehaviour.MouseMoveCommand="{Binding MenuButton.MouseEnterCommand}" u:MouseBehaviour.MouseLeaveCommand="{Binding MenuButton.MouseLeaveCommand}" u:MouseBehaviour.MouseDownCommand="{Binding MenuButton.MouseDownCommand}">
<Label Content="{Binding MenuButton.content}" Foreground="{Binding MenuButton.fontColor}" FontSize="{Binding MenuButton.fontSize}"/>
public class RootViewModel : ObservableObject
{
public MenuButtonViewModel MainPageButton { get; set; }
public MenuButtonViewModel SecondPageButton { get; set; }
public RootViewModel()
{
MainPageButton = new MenuButtonViewModel(new ButtonStyleA("Главная"));
SecondPageButton = new MenuButtonViewModel(new ButtonStyleB("Вторая"));
}
private void ChangeToMainPage()
{
}
private void ChangeToSecondPage()
{
}
}
private RootViewModel RootVM;
public MainWindow()
{
InitializeComponent();
RootVM = new RootViewModel();
this.DataContext = RootVM;
}
xmlns:bn="clr-namespace:WpfMvvmDemo.Views"
<Grid >
<StackPanel Orientation="Vertical">
<bn:MyButton DataContext="{Binding MainPageButton}" />
<bn:MyButton DataContext="{Binding SecondPageButton}"/>
</StackPanel>
</Grid>