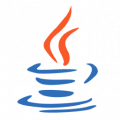
Java
- 1 ответ
- 0 вопросов
1
Вклад в тег
class Rect {
public int aX;
public int aY;
public int bX;
public int bY;
public int cX;
public int cY;
public int dY;
public int dX;
}
public Rect multiply(Rect source, float amount) {
Rect result = new Rect();
result.aX = source.aX * amount;
result.aY = source.aY * amount;
result.bX = source.bX * amount;
result.bY = source.bY * amount;
result.cX = source.cX * amount;
result.cY = source.cY * amount;
result.dX = source.dX * amount;
result.dY = source.dY * amount;
return result;
}
class Edge {
public float aX;
public float aY;
public float bX;
public float bY;
}
class Poly extends ArrayList<Edge> {
public Poly multiply(Poly source, float amount) {
Poly result = new Poly();
for (Edge edge : source) {
Edge newEdge = new Edge();
newEdge.aX = edge.aX * amount;
newEdge.aY = edge.aY * amount;
newEdge.bX = edge.bX * amount;
newEdge.bY = edge.bY * amount;
result.add(newEdge);
}
return result;
}
}
class Dot {
public float x;
public float y;
}
class Edge {
public Dot a;
public Dot b;
}
class Poly extends ArrayList<Dot> {
public List<Edge> edges = new ArrayList<>();
public Poly multiply(Poly source, float amount) {
Poly result = new Poly();
// Map just to remember old dots bindings
Map<Dot, Dot> newDots = new HashMap<>();
for (Dot dot : source) {
Dot newDot = new Dot();
newDot.x = dot.x * amount;
newDot.y = dot.y * amount;
result.add(dot);
newDots.put(dot, newDot);
}
for (Edge edge : edges) {
Edge newEdge = new Edge();
newEdge.a = newDots.get(edge.a);
newEdge.b = newDots.get(edge.b);
result.edges.add(newEdge);
}
return result;
}
}