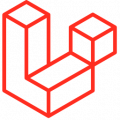
Laravel
- 18 ответов
- 0 вопросов
17
Вклад в тег
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Items extends Model
{
protected $fillable = ['name'];
public function categories() // название релейшена во Множественном числе, связь же ManyToMany
{
return $this->belongsToMany(
Category::class, // Название модели
'items_categories', // название твоей связующей таблицы
'item_id', // ключ к текущей таблице в связующей таблице
'category_id' // ключ к внешней таблице в связующей таблице
);
}
}
$item = Item::find($id)
$item->categories()->create($array_of_data)
/**
* Relations to Events
*
* @return HasMany
*/
public function events() : HasMany
{
return $this->hasMany(Events::class);
}
/**
* Relations to Places
*
* @return HasMany
*/
public function places() : HasMany
{
return $this->hasMany(Places::class);
}
$node = Node::create([
'title' => 'foobar',
'etc' => '123'
]);
$node->events()->create([
'title' => 'foobar',
'etc' => '123'
]);
$node->places()->create([
'title' => 'foobar',
'etc' => '123'
]);
Node::create([
'title' => 'foobar',
'etc' => '123'
])->events()->create([
'title' => 'foobar',
'etc' => '123'
]);