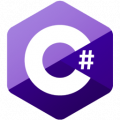
C#
- 14 ответов
- 0 вопросов
11
Вклад в тег
// To parse this JSON data, add NuGet 'Newtonsoft.Json' then do:
//
// using QuickType;
//
// var data = GettingStarted.FromJson(jsonString);
//
// For POCOs visit quicktype.io?poco
//
namespace QuickType
{
using System;
using System.Net;
using System.Collections.Generic;
using Newtonsoft.Json;
public partial class GettingStarted
{
[JsonProperty("measures")]
public Measures Measures { get; set; }
[JsonProperty("number2")]
public Number2 Number2 { get; set; }
[JsonProperty("items")]
public Items Items { get; set; }
[JsonProperty("number1")]
public Number1 Number1 { get; set; }
[JsonProperty("user")]
public User User { get; set; }
}
public partial class Measures
{
[JsonProperty("2")]
public OtherThe1 The2 { get; set; }
[JsonProperty("1")]
public OtherThe1 The1 { get; set; }
[JsonProperty("3")]
public OtherThe1 The3 { get; set; }
}
public partial class OtherThe1
{
[JsonProperty("name")]
public string Name { get; set; }
[JsonProperty("title")]
public string Title { get; set; }
}
public partial class Number2
{
[JsonProperty("2")]
public OtherOtherOtherThe1 The2 { get; set; }
[JsonProperty("1")]
public OtherOtherOtherThe1 The1 { get; set; }
[JsonProperty("3")]
public OtherOtherOtherThe1 The3 { get; set; }
}
public partial class OtherOtherOtherThe1
{
[JsonProperty("name")]
public string Name { get; set; }
[JsonProperty("description")]
public string Description { get; set; }
[JsonProperty("parent")]
public string Parent { get; set; }
[JsonProperty("title")]
public string Title { get; set; }
}
public partial class Items
{
[JsonProperty("2")]
public The1 The2 { get; set; }
[JsonProperty("1")]
public The1 The1 { get; set; }
[JsonProperty("3")]
public The1 The3 { get; set; }
}
public partial class The1
{
[JsonProperty("measure")]
public string Measure { get; set; }
[JsonProperty("number")]
public string Number { get; set; }
[JsonProperty("foxproID")]
public string FoxproID { get; set; }
[JsonProperty("name")]
public string Name { get; set; }
[JsonProperty("producer")]
public string Producer { get; set; }
[JsonProperty("suodID")]
public string SuodID { get; set; }
}
public partial class Number1
{
[JsonProperty("2")]
public OtherOtherThe1 The2 { get; set; }
[JsonProperty("1")]
public OtherOtherThe1 The1 { get; set; }
[JsonProperty("3")]
public OtherOtherThe1 The3 { get; set; }
}
public partial class OtherOtherThe1
{
[JsonProperty("description")]
public string Description { get; set; }
[JsonProperty("name")]
public string Name { get; set; }
}
public partial class User
{
[JsonProperty("login")]
public string Login { get; set; }
[JsonProperty("ip")]
public string Ip { get; set; }
[JsonProperty("number")]
public long Number { get; set; }
}
public partial class GettingStarted
{
public static GettingStarted FromJson(string json) => JsonConvert.DeserializeObject<GettingStarted>(json, Converter.Settings);
}
public static class Serialize
{
public static string ToJson(this GettingStarted self) => JsonConvert.SerializeObject(self, Converter.Settings);
}
public class Converter
{
public static readonly JsonSerializerSettings Settings = new JsonSerializerSettings
{
MetadataPropertyHandling = MetadataPropertyHandling.Ignore,
DateParseHandling = DateParseHandling.None,
};
}
}
public static class COM
{
public static SerialPort CurrentSerial {get; private set; }
public static InitSerialPort(string text, int baudRate)
{
CurrentSerial = new SerialPort();
CurrentSerial.PortName = text;
CurrentSerial.BaudRate = baudRate;
CurrentSerial.Open();
}
}
foreach (Process proc in Process.GetProcesses()) {
using (PerformanceCounter pcProcess = new PerformanceCounter("Process", "% Processor Time", proc.ProcessName)) {
pcProcess.NextValue();
System.Threading.Thread.Sleep(1000);
Console.WriteLine("Process:{0} CPU% {1}", proc.ProcessName, pcProcess.NextValue());
}
}