Можно так, мысль понятна, думаю, если и я правильно понял вопрос :)
Можно добавить и id родителя, если че.
Ну и методы в моделях, а не как тут :)
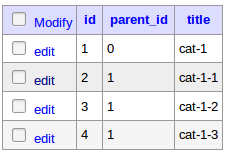
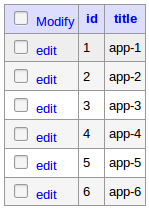

class Model_Category extends ORM
{
protected $_table_name = 'categories';
protected $_has_many = array
(
'subs' => array(
'model' => 'Category',
'foreign_key' => 'parent_id',
),
'apps' => array(
'model' => 'App',
'through' => 'categories_apps',
'foreign_key' => 'category_id',
'far_key' => 'app_id',
),
);
}
class Model_App extends ORM
{
protected $_table_name = 'apps';
protected $_has_many = array
(
'categories' => array(
'model' => 'Category',
'through' => 'categories_apps',
'foreign_key' => 'app_id',
'far_key' => 'category_id',
),
);
}
class Controller_Welcome extends Controller
{
public function action_index()
{
$id = 1;
// Variant 1
$subs_ids = $this->get_subs_ids_v1($id);
$this->show_subs_ids($subs_ids);
$this->show_apps($subs_ids);
// Variant 2
$subs_ids = $this->get_subs_ids_v2($id);
$this->show_subs_ids($subs_ids);
$this->show_apps($subs_ids);
}
private function get_subs_ids_v1($id)
{
// --------
// Category
$category = ORM::factory('Category', $id);
if ( ! $category->loaded())
throw new HTTP_Exception_404;
echo 'Category: ' . $category->title . '<br/>';
// ------------------
// Sub-Categories ids
return $category
->subs
->find_all()
->as_array(NULL, 'id');
}
private function get_subs_ids_v2($id)
{
// ------------------
// Sub-Categories ids
return ORM::factory('Category')
->where('parent_id', '=', $id)
->find_all()
->as_array(NULL, 'id');
}
private function show_subs_ids($subs_ids)
{
echo '<pre>' . print_r($subs_ids, TRUE) . '</pre>';
}
private function show_apps($subs_ids)
{
// $subs_ids может быть пустым, нужна дополнительная проверка,
// чтобы where IN не выдало шибку
$apps = ORM::factory('App')
->join('categories_apps')
->on('categories_apps.app_id', '=', 'app.id')
->where('categories_apps.category_id', 'IN', $subs_ids)
->find_all();
foreach ($apps as $app)
echo 'App: ' . $app->title . '<br/>';
}
}
Category: cat-1
Array
(
[0] => 2
[1] => 3
[2] => 4
)
App: app-1
App: app-2
App: app-3
App: app-4
App: app-5
App: app-6
Array
(
[0] => 2
[1] => 3
[2] => 4
)
App: app-1
App: app-2
App: app-3
App: app-4
App: app-5
App: app-6