У меня есть страница где находятся графики и таблицы. Данные приходят с бэка в таблицу и график.
В запросе сразу находятся данные для графика и для таблицы. Мне нужно как то обновлять именно таблицу, без графиков автоматически. Я не понимаю как это реализовать. Вот данные которые прилетают с бэка:
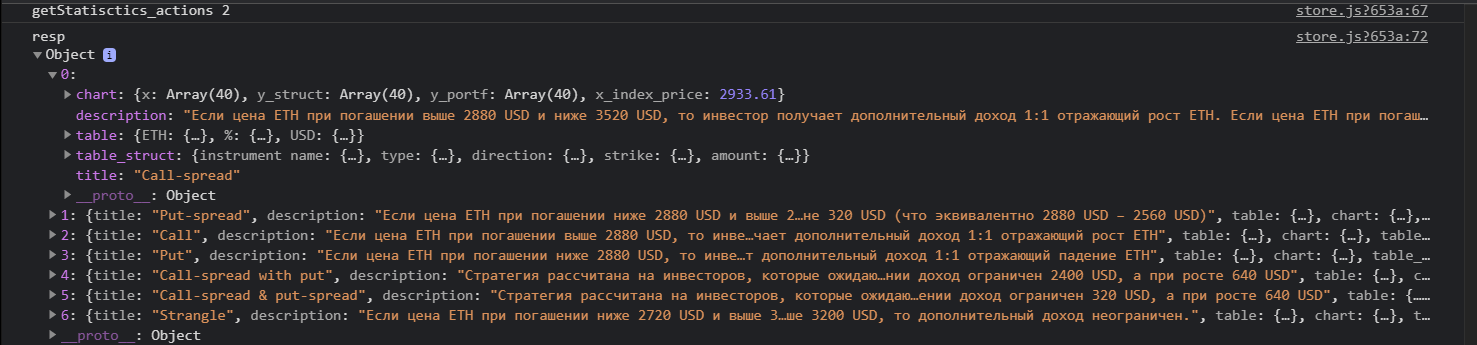
Мне нужно чтобы обновлялись данные в таблице которые лежат в ключе
table
Если я делаю
setInterval на весь
action то как раз обновление происходит и графиков и таблиц.
Как мне реализовать изменение данных в таблице?
store
import { createStore } from 'vuex'
import axios from 'axios'
let store = createStore({
state() {
return {
maturityList: [],
maturity: null,
underlying: null,
amount: 0,
futHedgeFlag: false,
fullDataList: null,
result: null,
value: null,
}
},
mutations: {
setMaturityList_mutations(state, maturityList) {
state.maturityList = maturityList
},
setMaturity_mutations(state, maturity) {
state.maturity = maturity
},
setUnderlying_mutations(state, underlying) {
state.underlying = underlying
},
setAmount_mutations(state, count) {
state.amount = count
console.log("teststtststs")
},
setFlagFutures_mutations(state, flag) {
state.futHedgeFlag = flag
console.log("flaggggggggggggg",flag)
},
setFullData_mutations(state, data) {
state.fullDataList = data
},
},
actions: {
getMaturity_actions({ commit }, underlying) {
return new Promise(( resolve, reject) => {
axios({ url: 'http://localhost:5000/maturities', params: {currency: underlying}, method: 'GET'})
.then(resp => {
const data = resp.data.data[underlying]
console.log("data", data)
commit('setMaturityList_mutations', data)
resolve(resp)
})
.catch(resp => {
reject(resp)
})
})
},
getStatisctics_actions({ commit }) {
console.log("getStatisctics_actions",this.state.amount)
return new Promise(( resolve, reject) => {
axios({ url: 'http://localhost:5000/recStructs', params: {currency: this.state.underlying, maturity: this.state.maturity, amount: this.state.amount, fut_hedge_flag: this.state.futHedgeFlag}, method: 'GET'})
.then(resp => {
commit('setFullData_mutations', resp.data.data) // Получаем все графики
console.log("resp",resp.data.data)
resolve(resp)
})
.catch(resp => {
reject(resp)
})
})
}
},
getters: {
maturityList: state => state.maturityList,
fullDataList: state => state.fullDataList,
underlyingChoice: state => state.underlying,
}
})
export default store;
v-table-statistics (компонент графика)
<template>
<div class="v-table-statistics flex justify-between w-full items-center">
<vChart class="text-left chart-v min-w-2/3 w-2/3" :dataset="chartData" :title="title" />
<div class="wrapper-text w-1/3 mt-20 ml-5 ">
<div class="text pr-3 ">{{description}} </div>
<table class="table-auto text-center mt-5 justify-end m-left">
<thead class="border border-gray-400 bg-gray-100">
<tr>
<th></th>
<th>{{ underlyingChoice }}</th>
<th>%</th>
<th>USD</th>
</tr>
</thead>
<tbody class="border border-gray-400">
<tr class="border-gray-400">
<td>Amount of underlying</td>
<td>{{ tableData [underlyingChoice] ['Amount of underlying'] }}</td>
<td>{{ tableData ['%'] ['Amount of underlying'] }}</td>
<td>{{ tableData ['USD'] ['Amount of underlying'] }}</td>
</tr>
<tr class="border-gray-400 bg-emerald-200">
<td>Max profit</td>
<td>{{ tableData [underlyingChoice] ["Max profit"].toFixed(2) }}</td>
<td>{{ tableData ['%'] ["Max profit"].toFixed(2)}}</td>
<td>{{ tableData ['USD'] ["Max profit"].toFixed(2) }}</td>
</tr>
<tr class="border-gray-400">
<td>Structure product price</td>
<td>{{ tableData [underlyingChoice] ["Structure product price"].toFixed(2) }}</td>
<td>{{ tableData ['%'] ["Structure product price"].toFixed(2) }}</td>
<td>{{ tableData ['USD'] ["Structure product price"].toFixed(2) }}</td>
</tr>
<tr class="border-gray-400">
<td>Maintenance margin</td>
<td>{{ tableData [underlyingChoice] ["Maintenace margin"].toFixed(2)}}</td>
<td>{{ tableData ['%'] ["Maintenace margin"].toFixed(2) }}</td>
<td>{{ tableData ['USD'] ["Maintenace margin"].toFixed(2) }}</td>
</tr>
<tr class="border-gray-400">
<td>Total margin</td>
<td>{{ tableData[underlyingChoice] ["Total margin"].toFixed(2) }}</td>
<td>{{ tableData ['%'] ["Total margin"].toFixed(2) }}</td>
<td>{{ tableData ['USD'] ["Total margin"].toFixed(2) }}</td>
</tr>
</tbody>
</table>
<div class="v-call-spread-right">
<vSendOrder @upGetStatisctics="getStatisctics_actions" />
</div>
</div>
</div>
</template>
<script>
import vSendOrder from "@/components/menu-right/forms/v-send-order"
import { mapGetters, mapActions } from 'vuex'
import vChart from "../v-vue-chart"
import watch from "vue"
export default {
components:{
vChart,
vSendOrder
},
name: "v-table-statistics",
props: {
tableData: {
type: Object,
default() {
return {}
}
},
description:{
type: String,
default() {
return ""
}
},
title:{
type: String,
default() {
return ""
}
},
chartData:{
type: Object,
default() {
return {}
}
}
},
computed: {
...mapGetters(["underlyingChoice","fullDataList", "chartData"])
},
watch: {
dataset: {
handler(newVal, oldVal) {},
immediate: true
},
logData() {
console.log("tableData",this.tableData)
},
},
methods: {
...mapActions(['getStatisctics_actions']),
},
data() {
return {};
},
};
</script>