При старте игры все объекты входят друг в друга и продолжают движение вместе, а должный двигаться друг за другом.
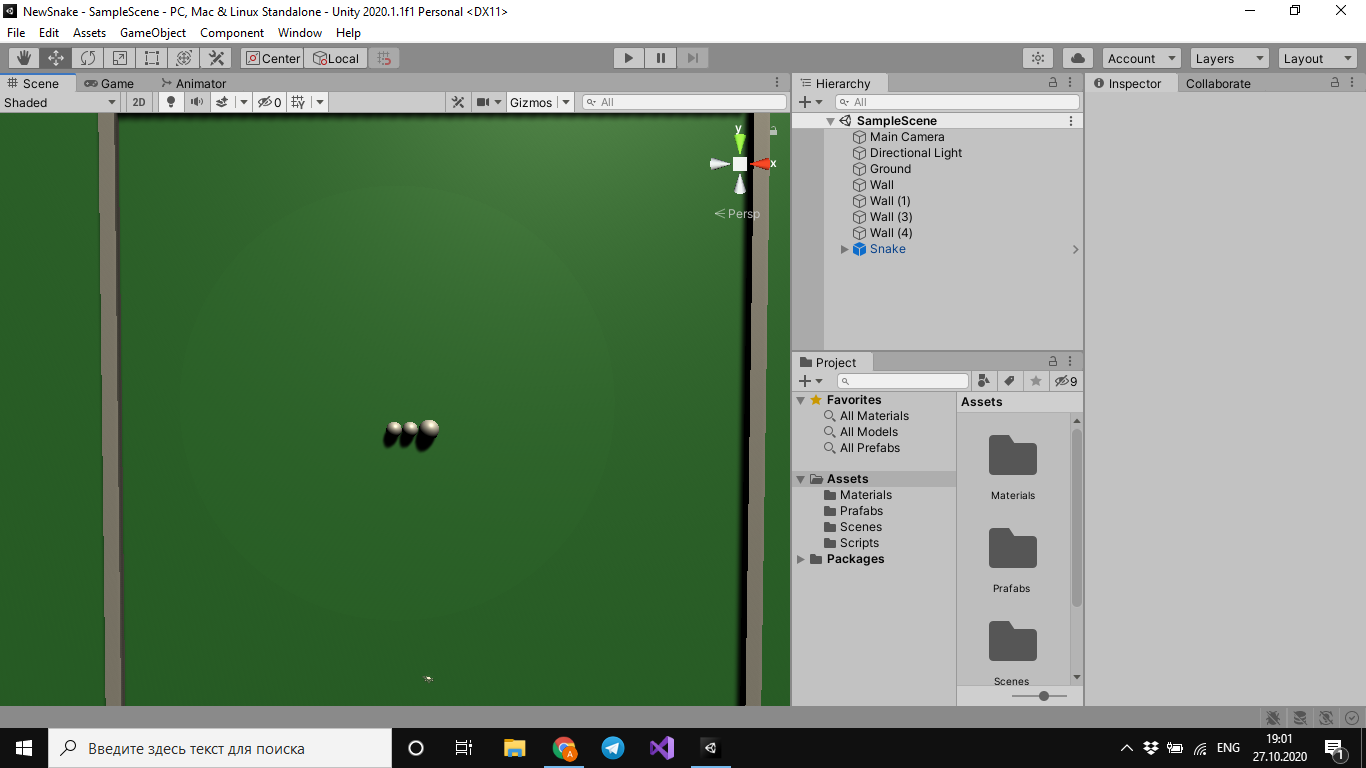
Код
using System.Collections;
using System.Collections.Generic;
using System.Xml.Linq;
using UnityEngine;
public class PlayerCintroller : MonoBehaviour
{
[HideInInspector]
public PlayerDirection direction;
[HideInInspector]
public float step_Length = 1f; //???
[HideInInspector]
public float movement_Frequensy = 0.2f;
private float counter;
private bool move;
[SerializeField]
private GameObject tailPrefab;
private List<Vector3> delta_Position;
private List<Rigidbody> nodes;
private Rigidbody main_Body;
private Rigidbody head_Body;
private Transform tr;
private bool createNodeAtTail;
void Awake()
{
tr = transform;
main_Body = GetComponent<Rigidbody>();
InitSnakeNodes();
InitPlayer();
delta_Position = new List<Vector3>
{
new Vector3(-step_Length,0f), //1
new Vector3(0f, step_Length), //2
new Vector3(step_Length, 0f), //3
new Vector3(0f, -step_Length),//4
};
}
void Update()
{
CheckMovementFrequency();
}
void FixedUpdate()
{
if (move)
{
move = false;
Move();
}
}
void InitSnakeNodes()
{
nodes = new List<Rigidbody>();
nodes.Add(tr.GetChild(0).GetComponent<Rigidbody>());
nodes.Add(tr.GetChild(1).GetComponent<Rigidbody>());
nodes.Add(tr.GetChild(2).GetComponent<Rigidbody>());
head_Body = nodes[0];
}
void SetDirectionRandom()
{
int dirRandom = Random.Range(0, (int)PlayerDirection.COUNT);
direction = (PlayerDirection)dirRandom;
}
void InitPlayer()
{
SetDirectionRandom();
switch (direction)
{
case PlayerDirection.RIGHT:
nodes[1].position = nodes[0].position - new Vector3(Metrics.NODE, 0f, 0f);
nodes[2].position = nodes[0].position - new Vector3(Metrics.NODE * 2f, 0f, 0f);
break;
case PlayerDirection.LEFT:
nodes[1].position = nodes[0].position + new Vector3(Metrics.NODE, 0f, 0f);
nodes[2].position = nodes[0].position + new Vector3(Metrics.NODE * 2f, 0f, 0f);
break;
case PlayerDirection.UP:
nodes[1].position = nodes[0].position - new Vector3( 0f, Metrics.NODE, 0f );
nodes[2].position = nodes[0].position - new Vector3( 0f, Metrics.NODE * 2f, 0f );
break;
case PlayerDirection.DOWN:
nodes[1].position = nodes[0].position + new Vector3(0f, Metrics.NODE, 0f);
nodes[2].position = nodes[0].position + new Vector3(0f, Metrics.NODE * 2f, 0f);
break;
}
}
void Move()
{
Vector3 dPosition = delta_Position[(int)direction];
Vector3 parentPosition = head_Body.position;
Vector3 prevPosition;
head_Body.position = head_Body.position + dPosition;
main_Body.position = main_Body.position + dPosition;
for (int i = 1; i < nodes.Count; i++)
{
prevPosition = nodes[i].position;
nodes[i].position = parentPosition;
parentPosition = prevPosition;
}
if (createNodeAtTail)
{
}
}
void CheckMovementFrequency()
{
counter += Time.deltaTime;
if (counter >= movement_Frequensy)
{
counter = 0f;
move = true;
}
}
}
Как решить, вопрос в коде или нужно настраивать в самом движке?