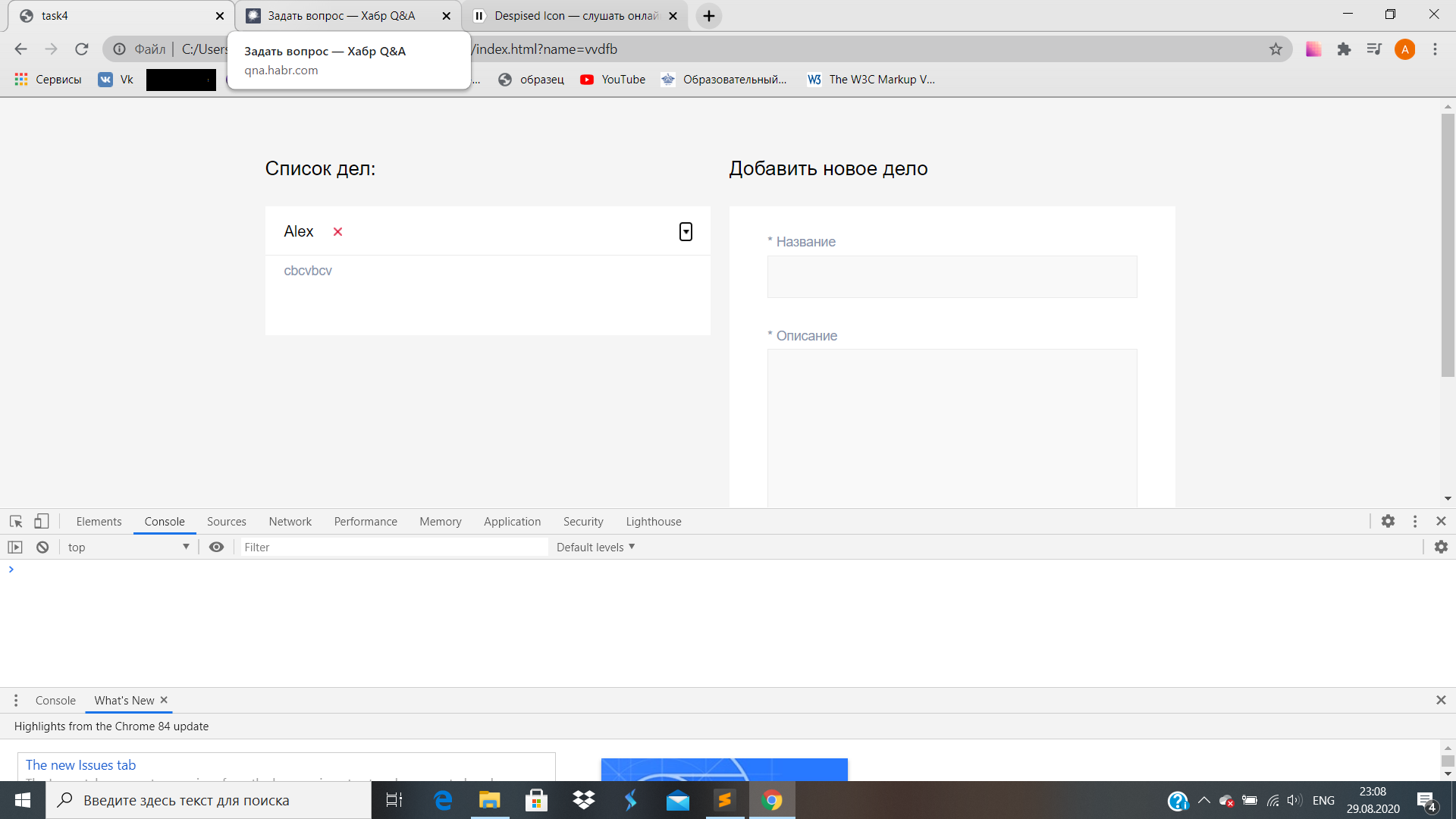
HTML
<!DOCTYPE html>
<html lang="ru">
<head>
<title>task4</title>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css" href="styles/normalize.css">
<link rel="stylesheet" type="text/css" href="styles/styles.css">
<link rel="stylesheet" type="text/css" href="styles/main.css">
<script src="scripts/jquery-3.4.1.min.js" defer></script>
<script src="scripts/btn.js" defer></script>
</head>
<body>
<div class="fixed-container clearfix">
<h1 class="visually-hidden">Список дел</h1>
<section>
<div class="case-list js-case-list clearfix">
<h2 class="title">Список дел:</h2>
<p class="emptyList js-empty-list">Список пуст...</p>
<ul class="to-do_list js-to-do_list"></ul>
</div>
</section>
<section>
<div class="addBlock clearfix">
<h2 class="title">Добавить новое дело</h2>
<form class="form js-form">
<div class="nameBlock">
<label class="inscription" for="name">* Название</label>
<input required class="name js-addName" id="name" type="text" name="name">
</div>
<div class="textBlock">
<label class="inscription" for="text">* Описание</label>
<textarea required class="text js-addText" id="text"></textarea>
</div>
<button type="submit" class="btn js-addTask">Добавить дело</button>
</form>
</div>
</section>
</div>
</body>
</html>
CSS
.title{
font-weight: 400;
font-size: 21px;
margin-bottom: 27px;
}
.nameTitle{
float: left;
width: 470px;
padding: 18px 20px 15px 20px;
margin-bottom: 8px;
border-bottom: 1px solid #ebebeb;
}
.newAdd{
margin: 0;
padding: 0;
}
.caseName{
float: left;
margin: 0 20px 0 0;
padding: 0;
font-size: 16px;
font-weight: 400;
}
.remove{
float: left;
border: none;
height: 18px;
width: 9px;
display: block;
background: url("../img/clear.png") no-repeat;
background-position: center;
cursor: pointer;
}
.slide{
float: right;
border: none;
height: 18px;
width: 9px;
display: block;
background: url("../img/стрелка.png") no-repeat;
background-position: center;
cursor: pointer;
transition: transform 0.5s;
}
.slide2{
float: right;
border: none;
height: 18px;
width: 9px;
display: block;
background: url("../img/стрелка2.png") no-repeat;
background-position: center;
cursor: pointer;
}
.caseText{
font-size: 14px;
color: #8993ad;
padding: 0 20px 45px 20px;
}
.to-do_list{
padding: 0;
}
.case{
list-style-type: none;
background-color: #fff;
float: left;
margin-bottom: 20px;
}
.case-list{
float: left;
width: 470px;
padding-bottom: 43px;
}
.emptyList{
color: #8993ad;
font-size: 16px;
margin-top: 72px;
}
.addBlock{
float: right;
width: 470px;
}
.form{
background-color: #fff;
width: 470px;
min-height: 505px;
padding: 30px 40px 0 40px;
}
.inscription{
font-size: 14px;
color: #8993ad;
font-weight: 400;
}
.name{
font-size: 16px;
font-weight: 400;
padding: 15px 14px 14px 14px;
margin-top: 5px;
background-color: #f9f9f9;
border: 1px solid #ebebeb;
width: 390px;
height: 45px;
}
.text{
font-size: 16px;
font-weight: 400;
padding: 21px 14px 14px 14px;
margin-top: 5px;
background-color: #f9f9f9;
border: 1px solid #ebebeb;
width: 390px;
height: 230px;
}
.btn{
display: inline-block;
margin-top: 5px;
border: none;
width: 224px;
height: 55px;
color: #fff;
font-size: 16px;
font-weight: 400;
background-color: #2174fd;
cursor: pointer;
}
.nameBlock{
margin-bottom: 32px;
}
.textBlock{
margin-bottom: 22px;
}
.rotate360 {
transition: transform 0.5s;
transform: rotate(90deg);
}
JQUERY
const addTaskEl = $(' .js-addTask');
const caseTitleEl = $(' .js-addName');
const caseTextEl = $(' .js-addText');
const caseListEl = $(' .js-case-list');
const toDoListEl = $(' .js-to-do_list');
const emptyListEl = $(' .js-empty-list');
const formEl = $(' .js-form');
formEl.on('submit', function(e) {
e.preventDefault();
const toDoCaseTitle = caseTitleEl.val();
const toDoCaseText = caseTextEl.val();
const newCase = (`
<li class='case js-addCase'>
<header class='nameTitle js-addNameTitle'>
<h2 class='caseName'> ${toDoCaseTitle} </h2>
<button type='button' aria-label='Удалить' class='remove js-remove'></button>
<button type='button' aria-label='Свернуть' class='slide js-slide'></button>
</header>
<p class='caseText'> ${toDoCaseText} </p>
</li>`);
toDoListEl.append(newCase);
emptyListEl.remove();
formEl.get(0).reset();
});
caseListEl.on('click', ' .js-remove', function(event){
$(this).closest(' .js-addCase').remove();
});
caseListEl.on('click', ' .js-slide', function(event){
$(this).toggleClass('rotate360');
$(this).closest(' .js-addNameTitle').siblings().slideToggle('slow');
//$(this).attr({'aria-label':'Развернуть'});
//alert($(this).attr('aria-label'));
});