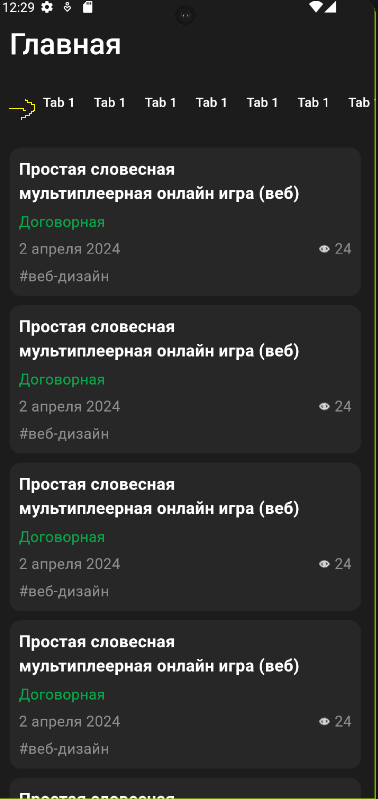
Приветствую, столкнулся с проблемой при изучении flutter. Пишу дипломную работу и нужно написать мобильное приложение, делаю главную страницу, на которой будет идти TabBar и он почему имеет какой-то странный margin, который я не могу убрать.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
const primaryColor = Color(0xFF0061FF);
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primaryColor: primaryColor,
scaffoldBackgroundColor: const Color(0xFF1C1C1C),
colorScheme: ColorScheme.fromSwatch(
primarySwatch: Colors.blue,
).copyWith(secondary: primaryColor),
useMaterial3: true,
),
home: const HomeScreen(),
);
}
}
class HomeScreen extends StatefulWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen>
with SingleTickerProviderStateMixin {
var _scrollController, _tabController;
@override
void initState() {
_scrollController = ScrollController();
_tabController = TabController(vsync: this, length: 7);
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: NestedScrollView(
controller: _scrollController,
headerSliverBuilder: (BuildContext context, bool innerBoxIsScrolled) {
return <Widget>[
SliverAppBar(
titleTextStyle: const TextStyle(
color: Colors.white,
fontSize: 32,
fontWeight: FontWeight.w500,
),
title: Text('Главная'),
backgroundColor: const Color(0xFF1C1C1C),
surfaceTintColor: const Color(0xFF1C1C1C),
pinned: true,
floating: true,
snap: false,
forceElevated: innerBoxIsScrolled,
bottom: PreferredSize(
preferredSize: Size.fromHeight(60),
child: Container(
width: double.infinity,
child: TabBar(
tabs: [
Tab(
child: Container(
margin: EdgeInsets.only(right: 20),
child: Text(
'Tab 1',
style: TextStyle(color: Colors.white),
),
),
),
Tab(
child: Container(
margin: EdgeInsets.only(left: 0, right: 20),
child: Text(
'Tab 1',
style: TextStyle(color: Colors.white),
),
),
),
Tab(
child: Container(
margin: EdgeInsets.only(right: 20),
child: Text(
'Tab 1',
style: TextStyle(color: Colors.white),
),
),
),
Tab(
child: Container(
margin: EdgeInsets.only(right: 20),
child: Text(
'Tab 1',
style: TextStyle(color: Colors.white),
),
),
),
Tab(
child: Container(
margin: EdgeInsets.only(right: 20),
child: Text(
'Tab 1',
style: TextStyle(color: Colors.white),
),
),
),
Tab(
child: Container(
margin: EdgeInsets.only(right: 20),
child: Text(
'Tab 1',
style: TextStyle(color: Colors.white),
),
),
),
Tab(
child: Container(
margin: EdgeInsets.only(right: 20),
child: Text(
'Tab 1',
style: TextStyle(color: Colors.white),
),
),
),
],
controller: _tabController,
isScrollable: true,
indicatorColor: Colors.transparent,
dividerColor: Colors.transparent,
indicatorPadding: EdgeInsets.zero,
indicatorSize: TabBarIndicatorSize.label,
labelPadding: EdgeInsets.zero),
),
)),
];
},
body: TabBarView(
controller: _tabController,
children: List.generate(
7,
(index) => _pageView(),
),
),
),
);
}
_pageView() {
return ListView.builder(
itemCount: 20,
itemBuilder: (BuildContext context, int index) {
return const HomeScreenItem();
},
);
}
}
class HomeScreenItem extends StatelessWidget {
const HomeScreenItem({
super.key,
});
@override
Widget build(BuildContext context) {
return Container(
height: 160,
padding: const EdgeInsets.symmetric(horizontal: 10, vertical: 10),
margin: const EdgeInsets.symmetric(horizontal: 16).copyWith(bottom: 10),
decoration: BoxDecoration(
color: const Color.fromRGBO(44, 44, 44, 100),
borderRadius: BorderRadius.circular(12)),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Container(
width: 310,
child: const Text(
'Простая словесная мультиплеерная онлайн игра (веб)',
style: TextStyle(
fontSize: 18,
color: Colors.white,
fontWeight: FontWeight.bold),
),
),
Container(
padding: const EdgeInsets.only(top: 6),
child: const Text(
'Договорная',
style: TextStyle(
fontSize: 16, color: Color.fromRGBO(0, 255, 97, 100)),
),
),
Padding(
padding: const EdgeInsets.only(top: 6),
child: Container(
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('2 апреля 2024',
style: TextStyle(
color: Color.fromRGBO(208, 208, 208, 100),
fontSize: 16)),
Row(
children: [
Image.asset('assets/images/view.png'),
const Padding(
padding: EdgeInsets.only(left: 5),
child: Text(
'24',
style: TextStyle(
color: Color.fromRGBO(208, 208, 208, 100),
fontSize: 16),
),
)
],
)
],
),
),
),
Container(
padding: const EdgeInsets.only(top: 6),
child: const Text(
'#веб-дизайн',
style: TextStyle(
fontSize: 16,
color: Color.fromRGBO(208, 208, 208, 100),
),
),
)
],
),
);
}
}