import random
import pygame
pygame.init()
# Задаем цвета
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
LIGHT_GRAY = (224, 224, 224)
FON = (230, 235, 210)
FPS = 60
#Высота и ширина
HEIGHT = 720
WIDTH = 1280
#Параметры
i = 0
j = 0
g = 0
k = True
minimum = 1468
food_list = [[300, 500]]
#X, Y
animal_list = [[500, 800, 400, BLUE, 10, 1], [500, 900, 200, BLUE, 10, 1]]
#Энергия, Х, Y, цвет, скорость, поиск еды(если 1 ищет, если 0 не ищет)
size = 10
# Создаем игру и окно
screen = pygame.display.set_mode((WIDTH, HEIGHT),pygame.RESIZABLE)
pygame.display.set_caption("PyGame")
bg_color = LIGHT_GRAY
clock = pygame.time.Clock()
class Animal():
'''Создает квадратики'''
#Размер квадаратиков
size = 10
def __init__(self, energy, animal_x, animal_y, color, speed, food_x, food_y):
self.animal_x = animal_x
self.animal_y = animal_y
self.food_x = food_x
self.food_y = food_y
self.energy = energy
self.speed = speed
self.color = color
def walking(self):
'''Перемещение'''
if ((self.animal_x != self.food_x) or (self.animal_y != self.food_y)) and (self.energy > 0):
if self.animal_x > self.food_x:
self.animal_x -= self.speed
self.energy -= 1
if abs(self.animal_x - self.food_x) < self.speed:
self.animal_x -= self.animal_x - self.food_x
if self.animal_x < self.food_x:
self.animal_x += self.speed
self.energy -= 1
if abs(self.animal_x - self.food_x) < self.speed:
self.animal_x += self.animal_x - self.food_x
if self.animal_y < self.food_y:
self.animal_y += self.speed
self.energy -= 1
if abs(self.animal_y - self.food_y) < self.speed:
self.animal_y += self.animal_y - self.food_y
if self.animal_y > self.food_y:
self.animal_y -= self.speed
self.energy -= 1
if abs(self.animal_y - self.food_y) < self.speed:
self.animal_y -= self.animal_y - self.food_y
pygame.draw.rect(screen, (self.color),
(self.animal_x, self.animal_y, size, size))
return [self.energy, self.animal_x, self.animal_y, self.color]
def generation(self):
'''Создание квадратиков'''
self.speed += random.randint(-1, 1)
return [1000, self.animal_x, self.animal_y, self.color, self.speed, 1000]
class Food():
'''Создает еду'''
def __init__(self):
pass
def generation():
food_x = random.randint(1, 1280)
food_y = random.randint(1, 720)
return [food_x, food_y]
while True:
screen.fill(FON)
#Чтобы окно закрывалось
for event in pygame.event.get():
if event.type == pygame.QUIT:
exit()
</spoiler>
#Движение квадратиков
while i < len(animal_list): #Перебор всех животных
if animal_list[i][5] == 1:
while g < len(food_list): #Перебор еды
if minimum > (abs(food_list[j][0]**2 + food_list[j][1]**2)**0.5):
minimum = (abs(food_list[j][0]**2 + food_list[j][1]**2))**0.5
food_x = food_list[j][0]
food_y = food_list[j][1]
g += 1
g = 0
a = Animal(animal_list[i][0], animal_list[i][1], animal_list[i][2], animal_list[i][3], animal_list[i][4], food_x, food_y)
animal_num = a.walking()
animal_list[i][0] = animal_num[0]
animal_list[i][1] = animal_num[1]
animal_list[i][2] = animal_num[2]
if animal_list[i][0] > 1500:
animal_list.append(a.generation())
animal_list[i][0] -= 1000
elif animal_list[i][0] <= 0:
del animal_list[i]
if len(animal_list) == 0:
animal_list.append([-1, 0, 0, 0, 0])
animal_list[i][5] = 0
i += 1
i = 0
# Еда
while i < len(animal_list):
while j < len(food_list):
if (animal_list[i][1] <= food_list[j][0]) and (animal_list[i][1] + size >= food_list[j][0]) and (animal_list[i][2] <= food_list[j][1]) and (animal_list[i][2] + size >= food_list[j][1]):
animal_list[i][0] += 300
animal_list[i][5] = 1
del food_list[j]
j += 1
i += 1
i = j = 0
if j % 240000 == 0:
s = Food.generation()
food_list.append(s)
k += 1
while i < len(food_list):
pygame.draw.rect(screen, (RED),
(food_list[i][0], food_list[i][1], 2, 2))
i += 1
i = 0
speed = 0
while i < len(animal_list):
pygame.draw.rect(screen, (BLUE),
(animal_list[i][1], animal_list[i][2], size, size))
speed += animal_list[i][4]
i += 1
sr_speed = speed//i
i = 0
#Текст
f1 = pygame.font.Font(None, 36)
populatoin = f1.render(str(len(animal_list)), True,
(0, 0, 0))
sr_speed_t = f1.render(str(sr_speed), True,
(0, 0, 0))
food_text = f1.render(str(len(food_list)), True,
(0, 0, 0))
screen.blit(populatoin, (10, 10))
screen.blit(sr_speed_t, (10, 40))
screen.blit(food_text, (10, 70))
pygame.display.flip()
clock.tick(FPS) #Количество кадров в секунду FPS 60
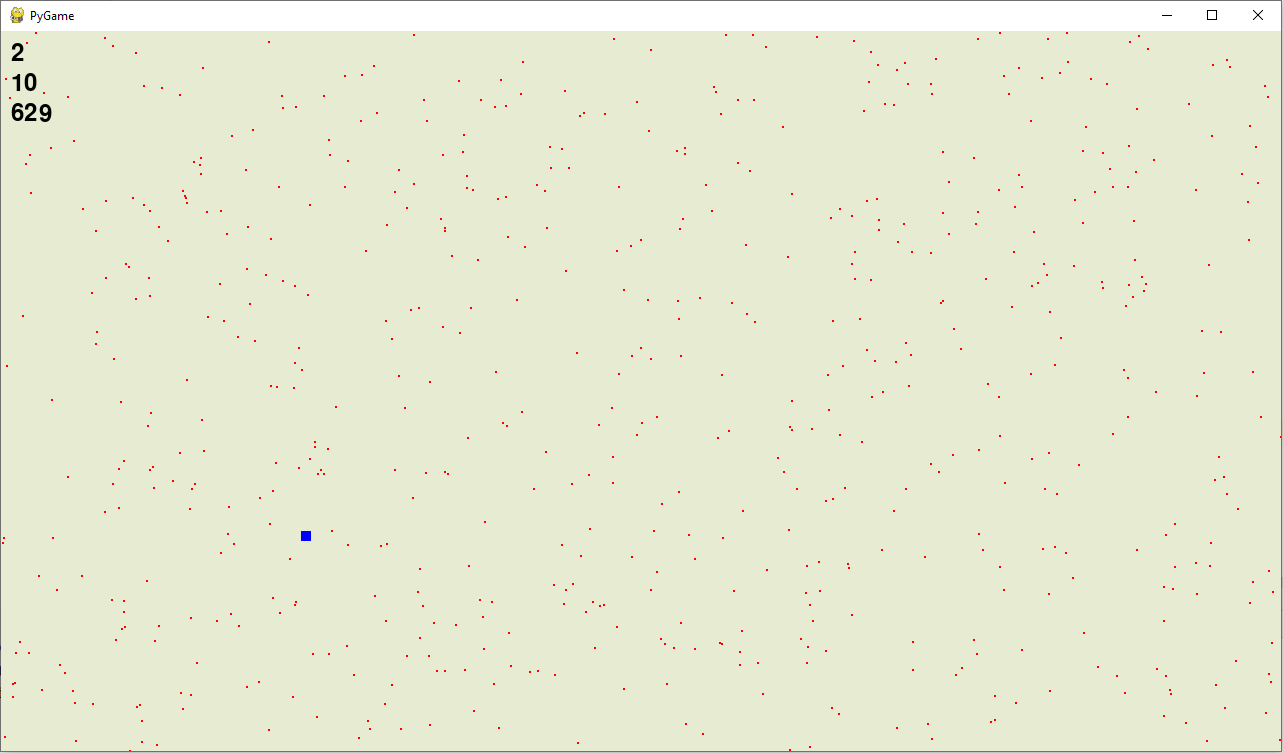