Есть самый обычный Todo проект, с кнопками удалить и обновить статус задачи.
Вопрос: как можно реализовать кнопку редактирование текста задачи?
screen:
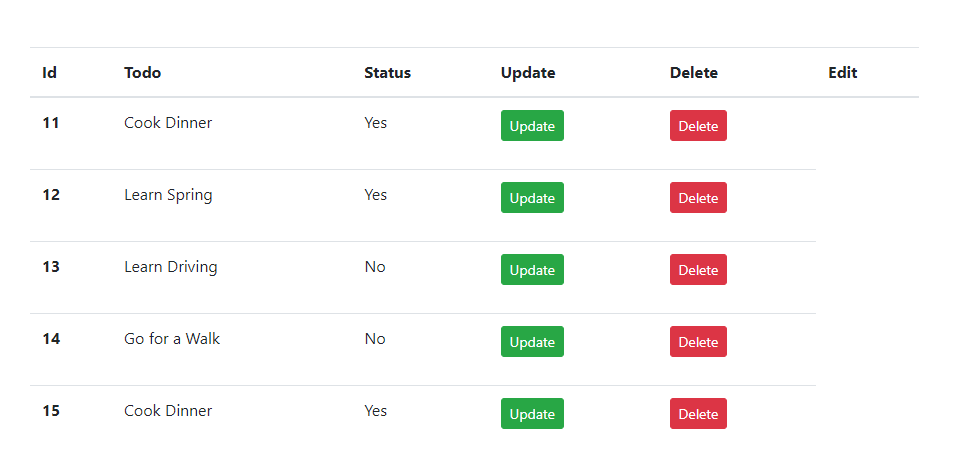
код контроллера:
@Controller
public class TodoController {
private TodoRepository todoRepository;
public TodoController(TodoRepository todoRepository) {
this.todoRepository = todoRepository;
}
@GetMapping
public String index(){
return "index";
}
@GetMapping("/todos")
public String todos(Model model){
model.addAttribute("todos", todoRepository.findAll());
return "todos";
}
@PostMapping("/todoNew")
public String add(@RequestParam String todoItem,
@RequestParam String status, Model model){
Todo todo = new Todo();
todo.setTodoItem(todoItem);
todo.setCompleted(status);
todoRepository.save(todo);
model.addAttribute("todos", todoRepository.findAll());
return "redirect:/todos";
}
@PostMapping("todoDelete/{id}")
public String delete(@PathVariable long id, Model model){
todoRepository.deleteById(id);
model.addAttribute("todos", todoRepository.findAll());
return "redirect:/todos";
}
@PostMapping("/todoUpdate/{id}")
public String update(@PathVariable long id, Model model){
Todo todo = todoRepository.findById(id).get();
if("Yes".equals(todo.getCompleted())) {
todo.setCompleted("No");
}
else {
todo.setCompleted("Yes");
}
todoRepository.save(todo);
model.addAttribute("todos", todoRepository.findAll());
return "redirect:/todos";
}
}
код thymeleaf:
<section id="todocontainer">
<div class="row">
<div class="col-md-2"></div>
<div class="col-md-8">
<table class="table">
<thead>
<tr>
<th scope="col">Id</th>
<th scope="col">Todo</th>
<th scope="col">Status</th>
<th scope="col">Update</th>
<th scope="col">Delete</th>
<th scope="col">Edit</th>
</tr>
</thead>
<tbody>
<tr th:each="todo : ${todos}">
<th scope="row" th:text=${todo.id}></th>
<td th:text=${todo.todoItem}></td>
<td th:text=${todo.completed}></td>
<td>
<form th:action="@{/todoUpdate/{id}(id=${todo.id})}" method="POST" enctype="multipart/form-data">
<div class="form-group">
<button type="submit" class="btn btn-success btn-sm text-white">Update</button>
</div>
</form>
</td>
<td>
<form th:action="@{/todoDelete/{id}(id=${todo.id})}" method="POST" enctype="multipart/form-data">
<div class="form-group">
<button type="submit" class="btn btn-danger btn-sm text-white">Delete</button>
</div>
</form>
</td>
</tr>
</tbody>
</table>
<div class="d-flex justify-content-center">
<a class="btn btn-success btn-lg text-white" data-toggle="modal" data-target="#viewModal">Add New Todo</a>
</div>
</div>
<div class="col-md-2"></div>
</div>
<!-- View Modal -->
<div class="modal fade" id="viewModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog modal-dialog-centered" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">TODO</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<form th:action="@{/todoNew}" method="POST" enctype="multipart/form-data">
<div class="form-group">
<label for="exampleInputEmail1">Todo</label>
<input type="text" class="form-control" name="todoItem" aria-describedby="emailHelp" placeholder="Enter Todo">
</div>
<div class="form-group">
<label for="exampleFormControlSelect1">Status</label>
<select class="form-control" name="status">
<option>Yes</option>
<option>No</option>
</select>
</div>
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary">Add Todo</button>
</form>
</div>
</div>
</div>
</div>
</section>