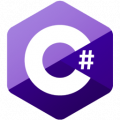
C#
- 3 ответа
- 0 вопросов
2
Вклад в тег
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Running;
using System.Security.Cryptography;
using System.Text.RegularExpressions;
namespace ConsoleApp19
{
[MemoryDiagnoser]
public partial class Program
{
const string whitlistchars = "qwertyuioplkjhgfdsazxcvbnmQWERTYUIOPLKJHGFDSAZXCVBNM1234567890-_";
static readonly string Source10k = string.Join("", Enumerable.Range(0, 10000).Select(x => whitlistchars[RandomNumberGenerator.GetInt32(0, whitlistchars.Length)]));
static readonly string Source128 = string.Join("", Enumerable.Range(0, 128).Select(x => whitlistchars[RandomNumberGenerator.GetInt32(0, whitlistchars.Length)]));
[GeneratedRegex("^[A-Za-z0-9_-]+$")] private static partial Regex Filter();
static readonly SortedSet<char> whiteList = new();
static bool CheckSymbolRegex(string symbol) => Filter().IsMatch(symbol);
static bool CheckSymbolNative(char symbol)
{
if ((symbol >= 'A' && symbol <= 'Z')
|| (symbol >= 'a' && symbol <= 'z')
|| (symbol >= '0' && symbol <= '9')
|| symbol == '_'
|| symbol == '-') return true;
return false;
}
[Benchmark]
public bool CheckRegex10k()
{
return CheckSymbolRegex(Source10k);
}
[Benchmark]
public bool CheckNative10k()
{
foreach (var symbol in Source10k)
{
if (!CheckSymbolNative(symbol)) return false;
}
return true;
}
[Benchmark]
public bool CheckSortedSet10k()
{
foreach (var symbol in Source10k)
{
if (!whiteList.Contains(symbol)) return false;
}
return true;
}
[Benchmark]
public bool CheckRegex128()
{
return CheckSymbolRegex(Source128);
}
[Benchmark]
public bool CheckNative128()
{
foreach (var symbol in Source128)
{
if (!CheckSymbolNative(symbol)) return false;
}
return true;
}
[Benchmark]
public bool CheckSortedSet128()
{
foreach (var symbol in Source128)
{
if (!whiteList.Contains(symbol)) return false;
}
return true;
}
static void InitWhiteList()
{
foreach (var symbol in whitlistchars)
{
whiteList.Add(symbol);
}
}
static void Main(string[] args)
{
InitWhiteList();
var summary = BenchmarkRunner.Run<Program>();
Console.ReadKey();
}
}
}