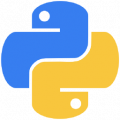
Python
- 24 ответа
- 0 вопросов
15
Вклад в тег
@commands.cooldown(1, (Время задержки), commands.BucketType.user)
async def on_command_error(ctx, error):
if isinstance(error, commands.CommandOnCooldown):
embed = discord.Embed(
title = 'Команда на задержке.',
description = f'Повторить через `{error.retry_after :.0f} секунд',
colour = discord.Color.red()
)
return await ctx.send(embed = embed)
import json
from discord import Embed
def get_embed(json_):
embed_json = json.loads(json_)
embed = Embed().from_dict(embed_json)
return embed
@bot.command(name = 'embed')
async def embed_send(ctx, channel: discord.TextChannel, *, args):
embed = get_embed(args)
if channel == "-":
await ctx.send(embed = embed)
else:
await channel.send(embed = embed)
from PIL import Image
@commands.command()
@commands.guild_only()
async def tweet(self, ctx, *, message):
api = nekobot.NekoBot()
message = str(api.tweet(ctx.author.display_name, message))[18:-59]
async with aiohttp.ClientSession() as ClientSession:
async with ClientSession.get(message) as reslink:
res = await reslink.read()
data = io.BytesIO(res)
image = Image.open(data)
file = io.BytesIO()
image.save(file, format="PNG")
file.seek(0)
embed = discord.Embed(color = 0x33FF57).set_image(url = "attachment://tweet.png")
await ctx.send(embed = embed, file = discord.File(image, filename="1.png"))
async def say(ctx, *, text):
def channel_conv(text):
value = text.split(" ")
string = value[0]
if string.isdigit():
return (
client.get_channel(int(string)),
text.replace(string, "")
)
elif string.startswith("<#"):
return (
client.get_channel(int(string[2:20])),
text.replace(string, "")
)
else:
return (
None,
text
)
try:
channel = channel_conv(text)
await channel[0].send(channel[1])
except:
await ctx.send(text)
from discord.ext.commands import TextChannelConverter
async def say(ctx, *, text):
v = text.split(" ")
try:
channel = await TextChannelConverter().convert(ctx = ctx, argument = v[0])
await channel.send(text.replace(v[0], ""))
except:
await ctx.send(text)