Доброго времени суток начал читать книгу, там некоторых фрагментов кода нет на githab исходники не нашёл к тому же на англ. Там написано что этот шаблон можно реализовать с помощью, как абстрактного класса так и интерфейса. Но из за интерфейса все методы публичные получаются когда пробую повысить до закрытого php начинает ругаться. Возможно я написал гомнокод так как автор не удосужился в книге полностью написать(
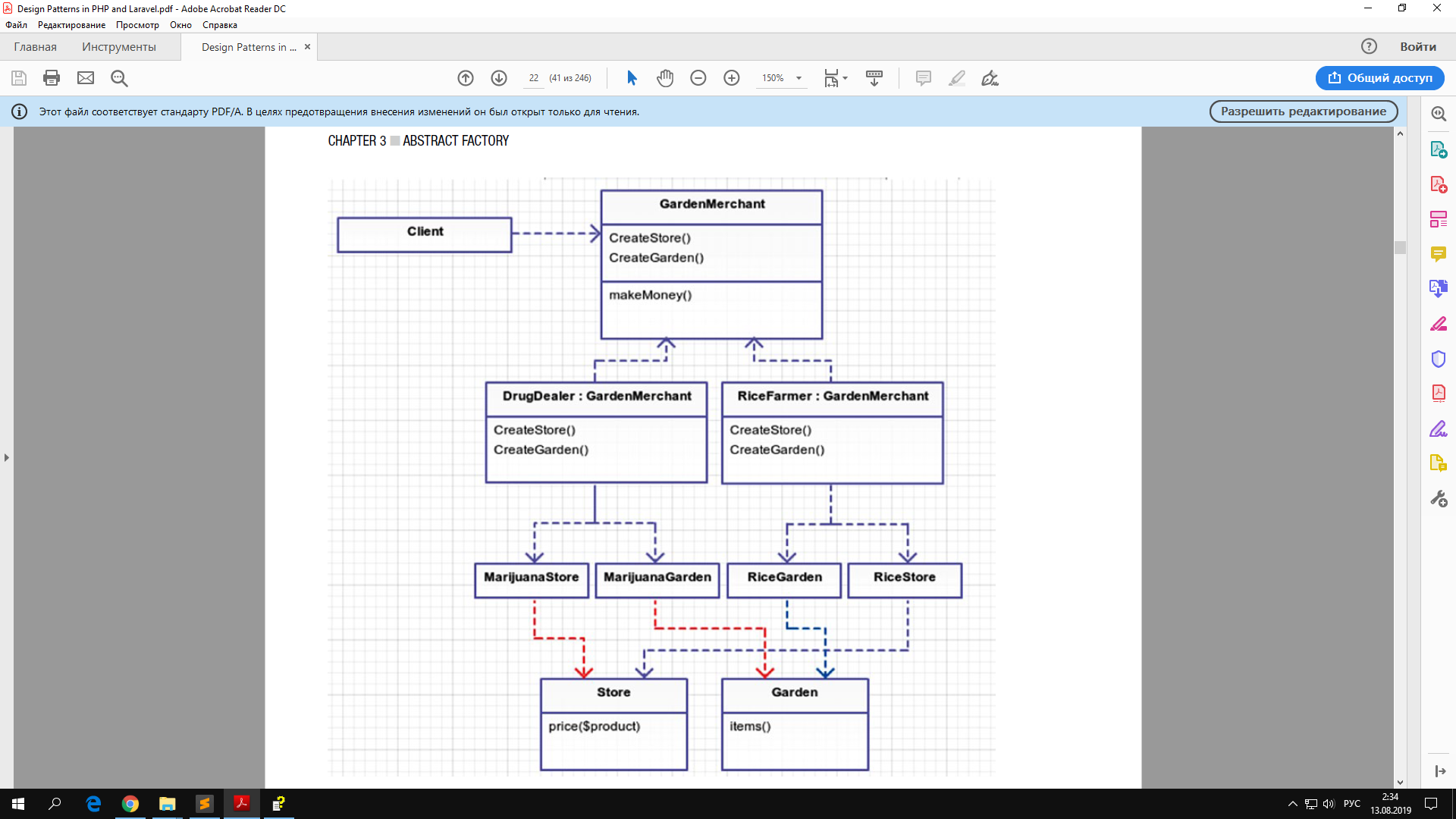
class Client
{
private $merchant;
public function __construct(GardenMerchant $merchant)
{
$this->merchant = $merchant;
}
public function run() : string
{
return 'Your merchant made $' . $this->merchant->makeMoney();
}
}
abstract class GardenMerchant
{
private $makeMoney = 0;
private $store;
private $items;
abstract protected function createStore();
abstract protected function createGarden();
public function makeMoney() : int
{
$this->store = $this->createStore();
$this->items = $this->createGarden()->items();
foreach ($this->items as $item)
{
$this->makeMoney += $this->store->price($item);
}
return $this->makeMoney;
}
}
class DrugDealer extends GardenMerchant
{
protected function createStore() : MarijuanaStore
{
return new MarijuanaStore;
}
protected function createGarden() : MarijuanaGarden
{
return new MarijuanaGarden;
}
}
class RiceFarmer extends GardenMerchant
{
protected function createStore() : RiceStore
{
return new RiceStore;
}
protected function createGarden() : RiceGarden
{
return new RiceGarden;
}
}
class MarijuanaStore
{
public function price(int $product) : int
{
return $product;
}
}
class RiceStore
{
public function price(int $product) : int
{
return $product;
}
}
class MarijuanaGarden
{
public function items() : array
{
return [300, 400, 500];
}
}
class RiceGarden
{
public function items() : array
{
return [100, 200, 300];
}
}
$drug_dealer = new DrugDealer();
$rice_farmer = new RiceFarmer();
$client1 = new Client($drug_dealer);
$client2 = new Client($rice_farmer);
echo $client1->run();
echo '<hr>';
echo $client2->run();
class Client
{
private $merchant;
public function __construct(GardenMerchantInterface $merchant)
{
$this->merchant = $merchant;
}
public function run()
{
echo 'Your merchant made $' . $this->merchant->makeMoney();
}
}
interface GardenMerchantInterface
{
public function createStore();
public function createGarden();
public function makeMoney();
}
trait MakeMoneyTrait
{
public function makeMoney()
{
$makeMoney = 0;
$store = $this->createStore();
$items = $this->createGarden()->items();
foreach ($items as $item)
{
$makeMoney += $store->price($item);
}
return $makeMoney;
}
}
class DrugDealer implements GardenMerchantInterface
{
use MakeMoneyTrait;
public function createStore()
{
return new MarijuanaStore;
}
public function createGarden()
{
return new MarijuanaGarden;
}
}
class RiceFarmer implements GardenMerchantInterface
{
use MakeMoneyTrait;
public function createStore()
{
return new RiceStore;
}
public function createGarden()
{
return new RiceGarden;
}
}
class MarijuanaStore
{
public function price($product)
{
return $product;
}
}
class RiceStore
{
public function price($product)
{
return $product;
}
}
class MarijuanaGarden
{
public function items()
{
return [300, 400, 500];
}
}
class RiceGarden
{
public function items()
{
return [100, 200, 300];
}
}
$drug_dealer = new DrugDealer();
$rice_farmer = new RiceFarmer();
$client1 = new Client($drug_dealer);
$client2 = new Client($rice_farmer);
$client1->run();
echo '<hr>';
$client2->run();