Код:
# coding=UTF-8
from asyncio import tasks
import discord
import os
from dislash import SlashClient, slash_command, Option, OptionType, OptionChoice, ResponseType, ActionRow, Button, ButtonStyle
from discord.ext import commands
import youtube_dl
import dislash
import asyncio
import functools
import itertools
import math
import random
import requests
import io
import json
import datetime
from asyncio import create_task, sleep
from async_timeout import timeout
import time
from PIL import Image, ImageFont, ImageDraw
from gtts import gTTS
#Половина кода стёрта т.к. она не касается темы проблемы
@slash_command(name="join",description="Подключить бота")
async def _join(self, ctx: commands.Context):
<spoiler title=""> """Подключается к голосовому каналу."""
ctx.voice_state = self.get_voice_state(ctx)
await ctx.reply(..., type=5)
destination = ctx.author.voice.channel
if ctx.voice_state.voice:
await ctx.voice_state.voice.move_to(destination)
return
ctx.voice_state.voice = await destination.connect()</spoiler>
@slash_command(name="stop", description="Остановить музыку")
async def _leave(self, ctx: commands.Context):
<spoiler title=""> """Очистить очередь и заставить бота уйти."""
ctx.voice_state = self.get_voice_state(ctx)
await ctx.reply(..., type=5)
if not ctx.voice_state.voice:
return await ctx.send('Бот и так не подключен. Зачем его кикать?')
await ctx.voice_state.stop()
await ctx.send('Пока!')
del self.voice_states[ctx.guild.id]</spoiler>
@slash_command(name="volume", description="Изменить громкости", options=[Option("volume","Укажите значение",required=True)])
async def _volume(self, ctx: commands.Context, *, volume = None):
<spoiler title=""> """Изменить громкость. Возможные значения(0-200)"""
ctx.voice_state = self.get_voice_state(ctx)
await ctx.reply(..., type=5)
if not volume:
return await ctx.reply('Использование команды:\n`mwb!volume <Значение от 5-200>`')
try:
volume = int(volume)
except ValueError:
return await ctx.reply(f'Эта команда вызвала исключение/ошибку: {volume} - Это не число!')
if not ctx.voice_state.is_playing:
return await ctx.send('Сейчас музыка не играет. Можете включить.')
if 0 > volume > 100:
return await ctx.send('Volume must be between 0 and 100')
ctx.voice_state.volume = volume / 100
await ctx.send('Громкость изменена на {}%, введите mwb!re'.format(volume))</spoiler>
@slash_command(name="now-playing",description="А что сейчас играет?")
async def _now(self, ctx: commands.Context):
<spoiler title=""> """Увидеть, какая песня играет прямо сейчас"""
ctx.voice_state = self.get_voice_state(ctx)
await ctx.reply(..., type=5)
if not ctx.voice_state.is_playing:
return await ctx.reply('Сейчас музыка просто не играет. Можешь включить.')
await ctx.send(embed=ctx.voice_state.current.create_embed())</spoiler>
@slash_command(name="skip", description="Пропустить трек")
async def _skip(self, ctx: commands.Context):
<spoiler title=""> ctx.voice_state = self.get_voice_state(ctx)
await ctx.reply(..., type=5)
if not ctx.voice_state.is_playing:
return await ctx.send('Сейчас музыка не играет,зачем её пропускать? Можете включить.')
if not ctx.voice_state.voice:
await ctx.invoke(self._join)
if (ctx.voice_state.current.requester):
ctx.voice_state.skip()
await ctx.reply(content=f'<:b654094678543279e1ff53713c1d65e7:956909939421499392>')</spoiler>
@slash_command(name="queue", description="Посмотреть очередь",options=[
Option("page", "Укажите страницу!", required=False)
# By default, Option is optional
# Pass required=True to make it a required arg
])
async def _queue(self, ctx: commands.Context, *, page: int = 1):
<spoiler title=""> ctx.voice_state = self.get_voice_state(ctx)
await ctx.reply(..., type=5)
if len(ctx.voice_state.songs) == 0:
return await ctx.send('В очереди нет треков. Можете добавить.')
items_per_page = 10
pages = math.ceil(len(ctx.voice_state.songs) / items_per_page)
start = (page - 1) * items_per_page
end = start + items_per_page
queue = ''
for i, song in enumerate(ctx.voice_state.songs[start:end], start=start):
queue += '`{0}.` [**{1.source.title}**]({1.source.url})\n'.format(i + 1, song)
embed = (discord.Embed(description='**{} tracks:**\n\n{}'.format(len(ctx.voice_state.songs), queue))
.set_footer(text='Viewing page {}/{}'.format(page, pages)))
await ctx.send(embed=embed)</spoiler>
@slash_command(name="shuffle", description="Перемешать очередь")
async def _shuffle(self, ctx: commands.Context):
<spoiler title=""> ctx.voice_state = self.get_voice_state(ctx)
await ctx.reply(..., type=5)
if len(ctx.voice_state.songs) == 0:
return await ctx.send('В очереди нет треков. Можете добавить.')
ctx.voice_state.songs.shuffle()
await ctx.message.add_reaction('✅')</spoiler>
@slash_command(name="remove", description="Удалить трек по индексу",options=[
Option("индекс", "Укажите индекс трека!", required=True)
# By default, Option is optional
# Pass required=True to make it a required arg
])
async def _remove(self, ctx: commands.Context, индекс = None):
<spoiler title=""> await ctx.reply(..., type=5)
ctx.voice_state = self.get_voice_state(ctx)
index = индекс
try:
index = int(index)
except ValueError:
return await ctx.reply(f'Эта команда вызвала исключение/ошибку: {index} - Это не индекс!')
if not index:
return await ctx.reply('Использование команды:\n`mwb!remove <Индекс>`')
if len(ctx.voice_state.songs) == 0:
return await ctx.send('В очереди нет треков. Можете добавить.')
ctx.voice_state.songs.remove(index - 1)
await ctx.message.add_reaction('✅')</spoiler>
@slash_command(name="play", description="Начать/Добавить трек",options=[
Option("название", "Введите название трека.", required=True)
# By default, Option is optional
# Pass required=True to make it a required arg
])
async def _play(self, ctx: commands.Context, *, название: str = None):
<spoiler title=""> await ctx.reply(..., type=5)
ctx.voice_state = self.get_voice_state(ctx)
search = название
if not search:
return await ctx.reply('Использование команды:\n`mwb!play <Ссылка, или название>`')
if not ctx.voice_state.voice:
destination = ctx.author.voice.channel
if ctx.voice_state.voice:
await ctx.voice_state.voice.move_to(destination)
return
ctx.voice_state.voice = await destination.connect()
msg = await ctx.reply(f'<a:19magnifierzoomsearchoutline:956909959709331488> Ожидаю ответ от `YouTube_DL`')
try:
source = await YTDLSource.create_source(ctx,
search,
loop=self.bot.loop)
except YTDLError as e:
await ctx.send('Ошибка: {}'.format(str(e)))
else:
song = Song(source)
await ctx.voice_state.songs.put(song)
await msg.edit(content=f'Добавлено {source}')</spoiler>
@slash_command(name="replay", description="Начать трек заново")
async def _re(self, ctx):
<spoiler title=""> ctx.voice_state = self.get_voice_state(ctx)
await ctx.reply(..., type=5)
if not ctx.voice_state.is_playing:
return await ctx.reply('Сейчас музыка не играет,зачем её реплеить? Можете включить.')
await ctx.reply(f'<a:19magnifierzoomsearchoutline:956909959709331488> Ожидаю ответ от `YouTube_DL`')
try:
source2 = await YTDLSource.create_source(ctx,
ctx.voice_state.current.now_name(),
loop=self.bot.loop)
except YTDLError as e:
await ctx.send('Ошибка: {}'.format(str(e)))
else:
song2 = Song(source2)
await ctx.voice_state.songs.put(song2)
ctx.voice_state.skip()
await ctx.reply(f'<:b654094678543279e1ff53713c1d65e7:956909939421499392>')</spoiler>
bot = commands.Bot(command_prefix="w!")
bot.add_cog(Music(bot))
bot.remove_command("help")
slash = SlashClient(bot)
@slash.command(name="help", description="Посмотреть список команд/View a list of commands")
async def _help(ctx):
embed = discord.Embed(title=f"Команды бота {bot.user.name}!",description="Их ещё нету ◕⌒◕")
await ctx.reply(embed=embed)
bot.run("194130951385932157")
Но ничего из этого не работает:
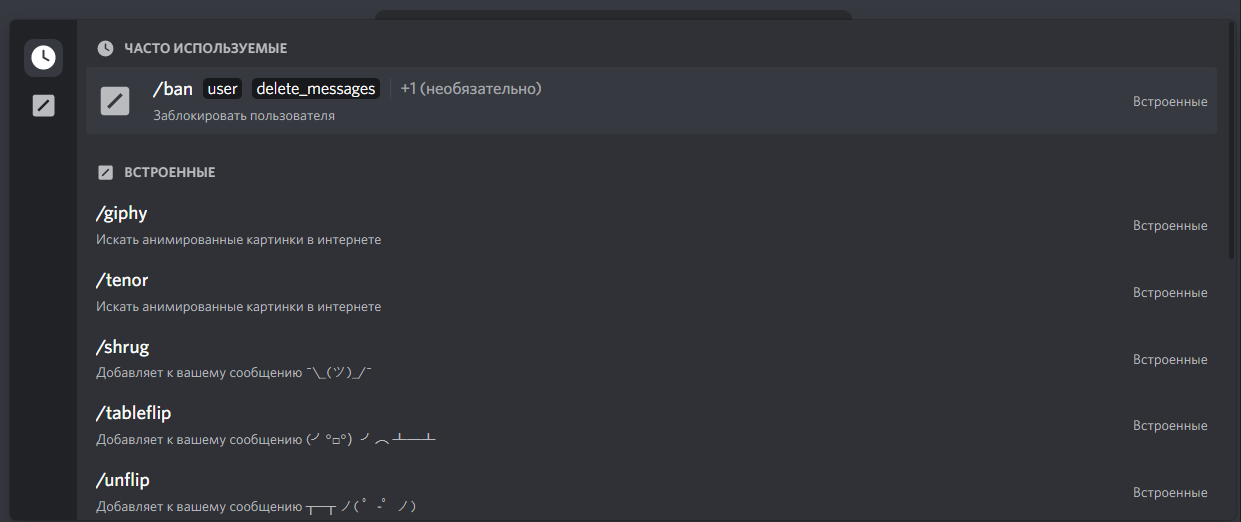
Уже бота преепригласить попробовал